Find the maximum of three integers
Calculate bike’s average consumption
Write a C program to calculate a bike’s average consumption from the given total distance (integer value) travelled (in km) and spent fuel (in litters, float number – 2 decimal points).
C Code:
#include <stdio.h>
int main()
{
int x; // Variable to store total distance in km
float y; // Variable to store total fuel spent in liters
// Prompt user for total distance and store in 'x'
printf("Input total distance in km: ");
scanf("%d",&x);
// Prompt user for total fuel spent and store in 'y'
printf("Input total fuel spent in liters: ");
scanf("%f", &y);
// Calculate and print average consumption
printf("Average consumption (km/lt) %.3f ",x/y);
printf("\n");
return 0;
}
Sample Output:
Input total distance in km: 350 Input total fuel spent in liters: 5 Average consumption (km/lt) 70.000
Flowchart:
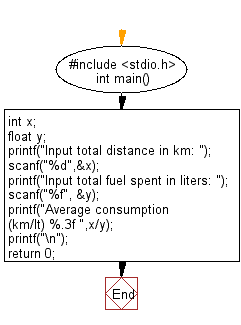
For more Practice: Solve these Related Problems:
- Write a C program to calculate fuel efficiency in miles per gallon, converting kilometers and liters appropriately.
- Write a C program to compute average fuel consumption for a bike when given distance traveled and fuel spent, ensuring input validation.
- Write a C program to calculate the remaining fuel after a journey given the initial fuel, distance, and consumption rate.
- Write a C program to compare fuel consumption rates of two bikes and determine which is more efficient.
C Programming Code Editor:
Previous: Write a C program that accepts three integers and find the maximum of three.
Next: Write a C program to calculate the distance between the two points.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.