Count positive and negative numbers in 5 inputs
C Practice Exercise
Write a C program that reads 5 numbers and counts the number of positive numbers and negative numbers.
Pictorial Presetation:
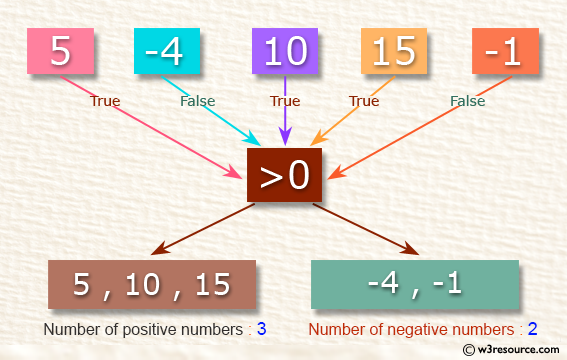
C Code:
#include <stdio.h>
int main() {
float numbers[5]; // Declare an array to store 5 numbers
int j, pctr=0, nctr=0; // Declare variables for loop counter, positive count, and negative count
// Prompt user for five numbers and store them in the array
printf("\nInput the first number: ");
scanf("%f", &numbers[0]);
printf("\nInput the second number: ");
scanf("%f", &numbers[1]);
printf("\nInput the third number: ");
scanf("%f", &numbers[2]);
printf("\nInput the fourth number: ");
scanf("%f", &numbers[3]);
printf("\nInput the fifth number: ");
scanf("%f", &numbers[4]);
for(j = 0; j < 5; j++) {
if(numbers[j] > 0) // Check if the number is positive
{
pctr++; // Increment positive count
}
else if(numbers[j] < 0) // Check if the number is negative
{
nctr++; // Increment negative count
}
}
// Print the counts of positive and negative numbers
printf("\nNumber of positive numbers: %d", pctr);
printf("\nNumber of negative numbers: %d", nctr);
printf("\n");
return 0;
}
Sample Output:
Input the first number: 5 Input the second number: -4 Input the third number: 10 Input the fourth number: 15 Input the fifth number: -1 Number of positive numbers: 3 Number of negative numbers: 2
Flowchart:
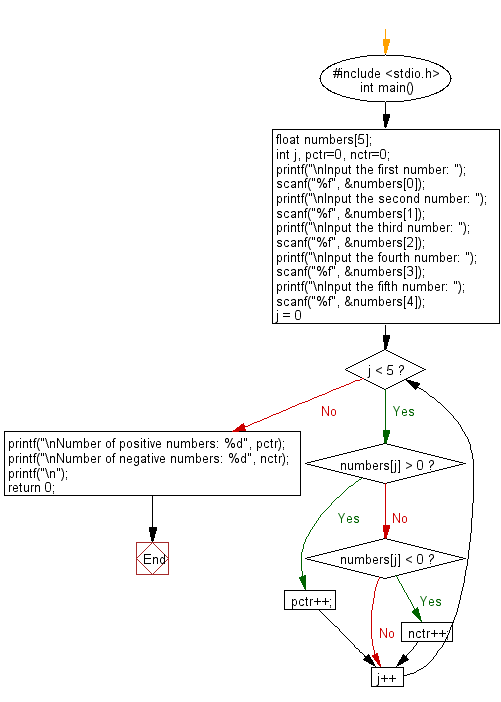
For more Practice: Solve these Related Problems:
- Write a C program to count the number of even and odd numbers among five inputs.
- Write a C program to count the positive, negative, and zero values from a set of five integers.
- Write a C program to count how many numbers in a list fall within a specified range.
- Write a C program to count the number of prime numbers among five integers entered by the user.
C Programming Code Editor:
Previous: Write a C program that prints all even numbers between 1 and 50 (inclusive).
Next: Write a C program that read 5 numbers and counts the number of positive numbers and print the average of all positive values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.