Determine quadrant of Cartesian coordinates (x, y)
C Practice Exercise
Write a C program to read the coordinates (x, y) (in the Cartesian system) and find the quadrant to which it belongs (Quadrant -I, Quadrant -II, Quadrant -III, Quadrant -IV).
Note: A Cartesian coordinate system is a coordinate system that specifies each point uniquely in a plane by a pair of numerical coordinates.
These are often numbered from 1st to 4th and denoted by Roman numerals: I (where the signs of the (x,y) coordinates are I(+,+), II (−,+), III (−,−), and IV (+,−).
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int x, y;
// Prompt for user input
printf("Input the Coordinate(x,y): ");
printf("\nx: ");
scanf("%d", &x);
printf("y: ");
scanf("%d", &y);
// Check which quadrant the point lies in
if(x > 0 && y > 0) {
printf("Quadrant-I(+,+)\n");
}
else if(x > 0 && y < 0) {
printf("Quadrant-II(+,-)\n");
}
else if(x < 0 && y < 0) {
printf("Quadrant-III(-,-)\n");
}
else if(x < 0 && y > 0) {
printf("Quadrant-IV(-,+)\n");
}
return 0;
}
Sample Output:
Input the Coordinate(x,y): x: 25 y: 15 Quadrant-I(+,+)
Flowchart:
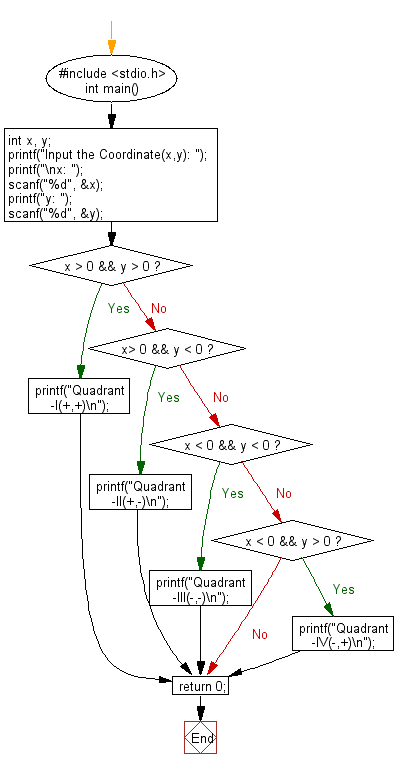
For more Practice: Solve these Related Problems:
- Write a C program to determine whether a given point lies on the X-axis, Y-axis, or in a quadrant.
- Write a C program to identify the quadrant of a point in a 2D Cartesian coordinate system and also check if it lies on the origin.
- Write a C program to determine the location of a point, indicating if it is on an axis, in a quadrant, or at the origin.
- Write a C program to calculate the distance of a point from the origin and then determine the quadrant in which the point lies.
C programming Code Editor:
Previous: Write a C program to read a password until it is correct. For wrong password print "Incorrect password" and for correct password print "Correct password" and quit the program. The correct password is 1234.
Next: Write a program that reads two numbers and divide the first number by second number. If the division not possible print "Division not possible".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.