Calculate the average of student math marks until termination
Calculate the average of student math marks until termination
Write a C program to calculate the average mathematics marks of some students. Input 0 (excluding to calculate the average) or a negative value to terminate the input process.
Pictorial Presentation:
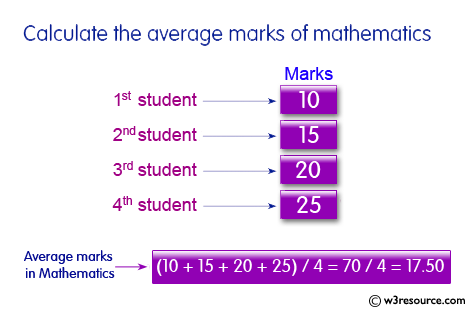
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int marks[99], m, i, a=0, total=0;
float f;
// Prompt for user input
printf("Input Mathematics marks (0 to terminate): ");
// Loop to get input marks
for(i = 0; ; i++) {
scanf("%d", &marks[i]);
if(marks[i] <= 0) {
break;
}
a++;
total += marks[i];
}
// Calculate and print average
f = (float)total/(float)a;
printf("Average marks in Mathematics: %.2f\n", f);
return 0;
}
Sample Output:
Input Mathematics marks (0 to terminate): 10 15 20 25 0 Average marks in Mathematics: 17.50
Flowchart:
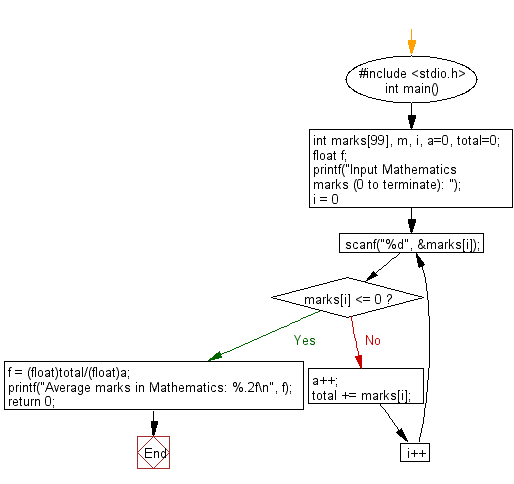
For more Practice: Solve these Related Problems:
- Write a C program to calculate the average marks for multiple subjects, terminating input when a negative value is entered.
- Write a C program to compute the average of student marks while ignoring invalid inputs (e.g., marks outside 0-100) until termination.
- Write a C program to continuously read student marks, count the number of pass and fail scores, and calculate the average for the passing marks only.
- Write a C program to dynamically calculate the average marks for an unspecified number of students, terminating on a specific sentinel value.
C programming Code Editor:
Previous: Write a C program that reads two integers p and q, print p number of lines in a sequence of 1 to b in a line.
Next: Write a C program to calculate the value of S where S = 1 + 1/2 + 1/3 + … + 1/50.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.