Find all divisors of a given integer
C Practice Exercise
Write a C program that reads and prints the elements of an array of length 7. Before printing, replace every negative number, zero, with 100.
Pictorial Presentation:
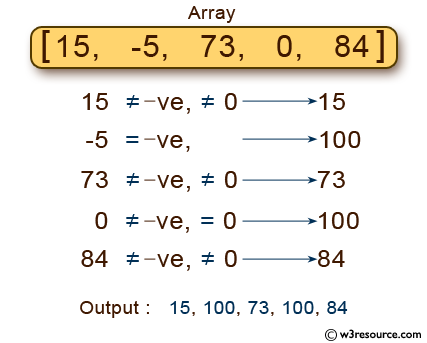
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int n[5], i, x;
// Input the 5 members of the array
printf("Input the 5 members of the array:\n");
for(i = 0; i < 5; i++) {
scanf("%d", &x);
if(x > 0) {
n[i] = x;
} else {
n[i] = 100;
}
}
// Print the array values
printf("Array values are: \n");
for(i = 0; i < 5; i++) {
printf("n[%d] = %d\n", i, n[i]);
}
return 0;
}
Sample Output:
Input the 5 members of the array: 25 45 35 65 15 Array values are: n[0] = 25 n[1] = 45 n[2] = 35 n[3] = 65 n[4] = 15
Flowchart:
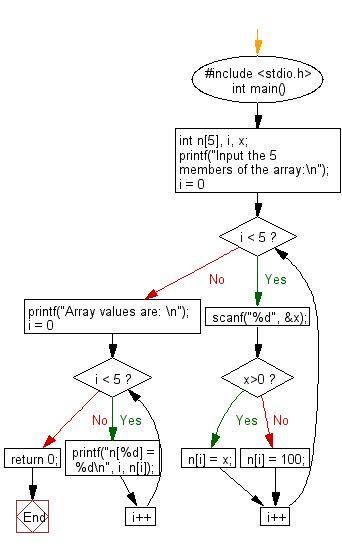
For more Practice: Solve these Related Problems:
- Write a C program to replace all even numbers in an array with a specified constant value.
- Write a C program to traverse an array and replace elements that are below a given threshold with the maximum element in the array.
- Write a C program to identify duplicate elements in an array and replace them with a sentinel value, such as -1.
- Write a C program to check each element in an array and, if it is a prime number, replace it with its square.
C programming Code Editor:
Previous: Write a C program that reads an integer and find all its divisor.
Next: Write a C program to read and print the elements of an array of length 7, before print, put the triple of the previous position starting from the second position of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.