Print positions and values of elements in an array < 5
C Practice Exercise
Write a C program to read an array of length 5 and print the position and value of the array elements of value less than 5.
Pictorial Presentation:
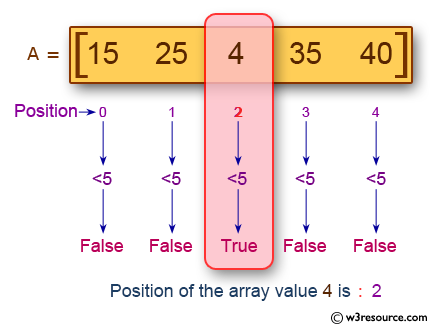
Sample Solution:
C Code:
#include <stdio.h>
#define AL 5 // Define the size of the array as 5
#define MAX 5 // Define a maximum value for comparison
int main() {
float N[AL]; // Declare an array of size 5 to hold floating-point numbers
int i;
// Prompt for user input
printf("Input the 5 members of the array:\n");
// Read and store user input into the array
for(i = 0; i < AL; i++) {
scanf("%f", &N[i]);
}
// Iterate through the array and print elements less than MAX
for(i = 0; i < AL; i++) {
if(N[i] < MAX) {
printf("A[%d] = %.1f\n", i, N[i]);
}
}
return 0;
}
Sample Output:
Input the 5 members of the array: 15 25 4 35 40 A[2] = 4.0
Flowchart:
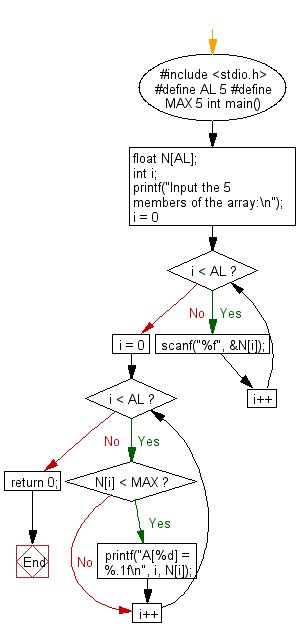
For more Practice: Solve these Related Problems:
- Write a C program to print the positions and values of array elements that are greater than 10.
- Write a C program to identify and print the indices and values of even numbers in an array.
- Write a C program to find and display the positions and values of prime numbers within an array.
- Write a C program to print the positions and values of elements in an array that are perfect squares.
C programming Code Editor:
Previous: Write a C program to read and print the elements of an array of length 7, before print, put the triple of the previous position starting from the second position of the array.
Next: Write a C program to read an array of length 6, change the first element by the last, the second element by the fifth and the third element by the fourth. Print the elements of the modified array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.