C Exercises: Shift the specified data by two bits to the left
Left-shift an integer by two bits
Write a C program to shift given data by two bits to the left.
Sample Solution:
C Code:
#include<stdio.h>
int main() {
int a, b;
// Prompt user to input an integer
printf("Read the integer from keyboard-");
scanf("%d",&a);
// Display the original integer value
printf("\nInteger value = %d ",a);
// Left shift 'a' by 2 bits and assign it to 'b'
a <<= 2;
b = a;
// Display the left shifted data
printf("\nThe left shifted data is = %d ",b);
return 0;
}
Sample Output:
Read the integer from keyboard- Integer value = 2 The left shifted data is = 8
Flowchart:
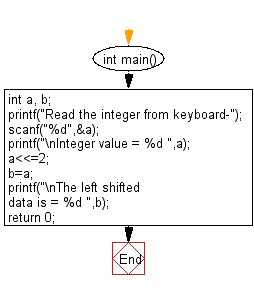
For more Practice: Solve these Related Problems:
- Write a C program to perform a left bitwise shift on an integer by a user-specified number of bits.
- Write a C program to demonstrate left shifting on negative integers and observe the effects.
- Write a C program to perform a circular left shift on an integer’s binary representation.
- Write a C program to combine left and right shifts to swap halves of an integer’s bit pattern.
C programming Code Editor:
Previous: Write a C program that swaps two numbers without using third variable.
Next: Write a C program to reverse and print a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.