C Exercises: Accepts a positive integer n less than 100 from the user and prints out the sum
C Basic Declarations and Expressions: Exercise-63 with Solution
Sum the series 1^4+2^4+4^4+...+m^4
Write a C program that accepts a positive integer n less than 100 from the user. It prints out the sum of 14 + 24 + 44 + 74 + 114 + • • • + m4. In this case, m is less than or equal to n. Print an appropriate message.
Test data and expected output:
Input a positive number less than 100:
Sum of the series is 1024388
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int i, j, n, sum_int = 0;
// Prompt user for input
printf("Input a positive number less than 100: \n");
// Read the input value
scanf("%d", &n);
// Check if the input is valid
if (n < 1 || n >= 100) {
printf("Wrong input\n");
return 0;
}
j = 1;
for (i = 1; j <= n; i++) {
sum_int += j * j * j * j;
j += i;
}
// Display the result
printf("Sum of the series is %d\n", sum_int);
return 0;
}
Sample Output:
Input a positive number less than 100: 68 Sum of the series is 37361622
Flowchart:
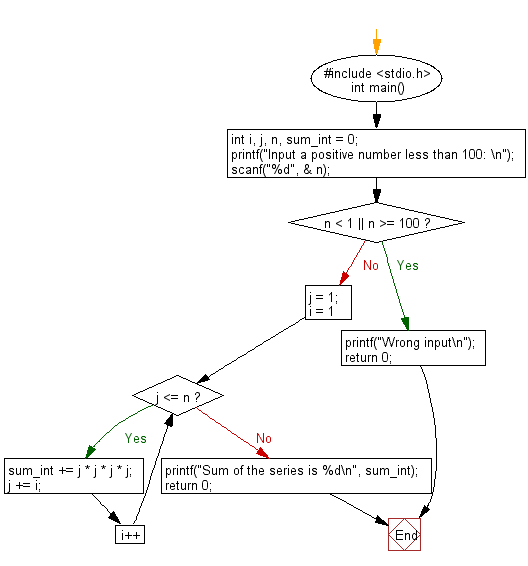
C programming Code Editor:
Previous:Write a C program that accepts a positive integer less than 500 and prints out the sum of the digits of this number.
Next: Write a C program that accepts integers from the user until a zero or a negative number, display the number of positive values, the minimum value, the maximum value and the average of all numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-declarations-and-expressions/c-programming-basic-exercises-63.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics