C Exercises: Demonstrates the difference between predecrementing and postdecrementing using the decrement operator
Demonstrate predecrementing vs postdecrementing
Write a C program to demonstrate the difference between predecrementing and postdecrementing using the decrement operator --.
Sample Solution:
C Code:
#include<stdio.h>
int main()
{
int x = 10; // Initialize variable x with value 10
// Predecrementing
printf("Predecrementing:\n");
printf("x = %d\n", x); // Print current value of x
printf("x-- = %d\n", x--); // Print x and then decrement it
printf("x = %d\n\n", x); // Print updated value of x
x = 10; // Reset x to 10
// Postdecrementing
printf("Postdecrementing:\n");
printf(" x = %d\n", x); // Print current value of x
printf("--x = %d\n", --x); // Decrement x and then print it
printf(" x = %d\n", x); // Print updated value of x
return 0;
}
Sample Output:
Predecrementing: x = 10 x-- = 10 x = 9
Flowchart:
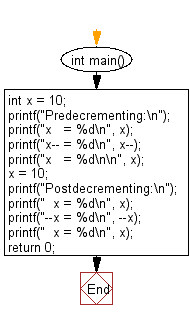
For more Practice: Solve these Related Problems:
- Write a C program to illustrate the differences between predecrement and postdecrement in various expressions.
- Write a C program to demonstrate the effects of pre- and post-decrement operations within loop constructs.
- Write a C program to show how predecrement and postdecrement affect pointer arithmetic in an array.
- Write a C program to compare the results of predecrement vs postdecrement in complex arithmetic expressions.
C programming Code Editor:
Previous:Write a C program that accepts principal amount, rate of interest and days for a loan and calculate the simple interest for the loan, using the following formula.
Next: Write a C program using looping to produce the following table of values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.