C Exercises: Reads in a five-digit integer and determines whether or not it’s a palindrome
Check if a 5-digit integer is a palindrome
Write a C program that reads a five-digit integer and determines whether or not it's a palindrome.
Sample Input: 33333
Sample Solution:
C Code:
#include <stdio.h>
// Function to check if a number is a palindrome
int is_Palindrome(int);
int main()
{
int n;
// Prompt user for input
printf("Input a five-digit number: ");
// Read the number from user
scanf("%d", &n);
// Check if the number is a palindrome using the function is_Palindrome
if (is_Palindrome(n))
printf("%d is a palindrome.", n);
else
printf("%d is not a palindrome.", n);
return 0;
}
// Function to check if a number is a palindrome
int is_Palindrome(int n) {
int x = n;
int reverse_num = 0;
// Extract the digits in reverse order and reconstruct the number
reverse_num += x / 10000;
x = x - ((x / 10000) * 10000);
reverse_num += ((x / 1000) * 10);
x = x - ((x / 1000) * 1000);
reverse_num += ((x / 100) * 100);
x = x - ((x / 100) * 100);
reverse_num += ((x / 10) * 1000);
x = x - ((x / 10) * 10);
reverse_num += ((x % 10) * 10000);
// Check if the original number is equal to the reversed number
return n == reverse_num;
}
Sample Output:
Input a five-digit number: 33333 is a palindrome.
Pictorial Presentation:
Flowchart:
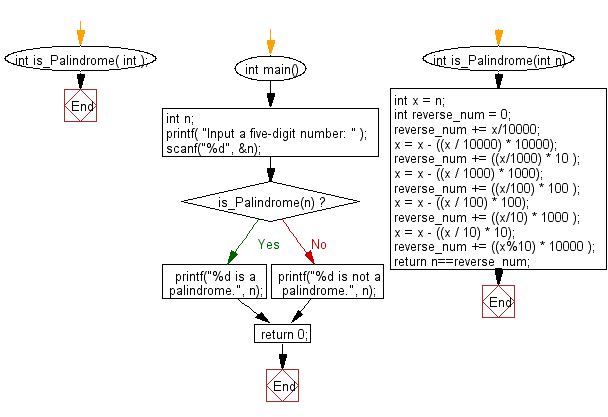
For more Practice: Solve these Related Problems:
- Write a C program to check if a 5-digit integer is a palindrome without converting it to a string.
- Write a C program to verify a 5-digit number’s palindrome property using mathematical operations.
- Write a C program to determine if a 5-digit integer is a palindrome and display its reverse.
- Write a C program to check for a palindrome in a 5-digit number using an iterative loop and modulus.
C programming Code Editor:
Previous:Write a C program that reads the side (side sizes between 1 and 10 ) of a square and prints a hollow square using hash (#) character.
Next: Write a C program which reads an integer (7 digits or fewer) and count number of 3s in the given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.