C Exercises: Convert a tm object to custom wide string textual representation
C Date Time: Exercise-5 with Solution
Write a program in C to convert a tm object to a custom wide string textual representation.
Sample Solution:
C Code:
#include <stdio.h>
#include <time.h>
#include <wchar.h>
#include <locale.h>
int main(void) {
wchar_t buff[40]; // Wide character array to store the formatted date and time string
// Create a custom time structure with specified date and time
struct tm mytime = {
.tm_year = 116, // = year 2016 (years since 1900)
.tm_mon = 8, // = 9th month (0-based index)
.tm_mday = 2, // = 2nd day of the month
.tm_hour = 17, // = 17 hours
.tm_min = 51, // = 51 minutes
.tm_sec = 10 // = 10 seconds
};
printf("\n The textual representation of specified date and time :\n");
// Format the time structure to a wide character string based on the provided format specifier
if (wcsftime(buff, sizeof buff, L"%A %c", &mytime)) {
printf("\n%ls\n", buff); // Print the formatted date and time string using wide character format
} else {
puts("wcsftime failed"); // Print a message if wcsftime fails
}
setlocale(LC_ALL, "en_US.UTF-8"); // Change the locale to English (US) in UTF-8 encoding
if (wcsftime(buff, sizeof buff, L"%A %c", &mytime)) {
printf("%ls\n\n", buff); // Print the formatted date and time string in the specified locale
} else {
puts("wcsftime failed"); // Print a message if wcsftime fails after changing the locale
}
return 0;
}
Sample Output:
The textual representation of specified date and time : Sunday 09/02/16 17:51:10 Sunday 09/02/16 17:51:10
Flowchart:
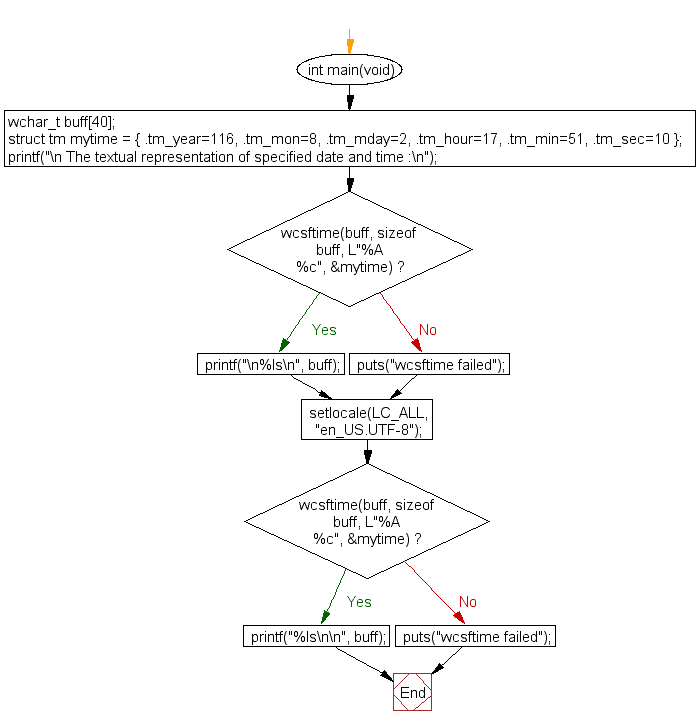
C Programming Code Editor:
Previous: Write a program in C to convert a tm object to custom textual representation.
Next: Write a program in C to convert a time_t object to calendar time expressed as Coordinated Universal Time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/datetime/c-datetime-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics