C Program: Guess the number game with Do-While Loop
C Do-While Loop: Exercise-5 with Solution
Write a C program that generates a random number between 1 and 100 and asks the user to guess it. Use a do-while loop to give the user multiple chances until they guess the correct number.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int randomNumber, userGuess, attempts = 0;
// Seed the random number generator based on current time
srand(time(NULL));
// Generate a random number between 1 and 100
randomNumber = rand() % 10 + 1;
// Welcome message
printf("Welcome to the Guess the Number Game!\n");
// Guessing loop
do {
// Prompt the user to enter a guess
printf("Input your guess (between 1 and 10): ");
scanf("%d", &userGuess);
// Increment the number of attempts
attempts++;
// Check if the guess is correct
if (userGuess == randomNumber) {
printf("Congratulations! You guessed the correct number in %d attempts.\n", attempts);
} else if (userGuess < randomNumber) {
printf("Number is too low. Try again!\n");
} else {
printf("Number is too high. Try again!\n");
}
} while (userGuess != randomNumber); // Continue the loop until the user guesses the correct number
return 0;
}
Sample Output:
Welcome to the Guess the Number Game! Input your guess (between 1 and 10): 2 Number is too high. Try again! Input your guess (between 1 and 10): 3 Number is too high. Try again! Input your guess (between 1 and 10): 1 Congratulations! You guessed the correct number in 3 attempts.
Explanation:
Here are key parts of the above code step by step:
- int randomNumber, userGuess, attempts = 0;: Declares variables to store the randomly generated number, the user's guess, and the number of attempts.
- srand(time(NULL));: Seeds the random number generator based on the current time to ensure a different random number on each program run.
- randomNumber = rand() % 100 + 1;: Generates a random number between 1 and 100.
- printf("Welcome to the Guess the Number Game!\n");: Displays a welcome message.
- do {: Marks the beginning of a do-while loop. The code inside the loop will be executed at least once before checking the loop condition.
- printf("Enter your guess (between 1 and 100): ");: Prompts the user to input a guess.
- scanf("%d", &userGuess);: Reads the user's guess.
- attempts++;: Increments the number of attempts.
- Checks if the guess is correct and provides feedback to the user.
- } while (userGuess != randomNumber);: Specifies the loop condition. The loop continues until the user guesses the correct number.
- return 0;: Indicates successful program execution.
- }: Marks the end of the "main()" function.
Flowchart:
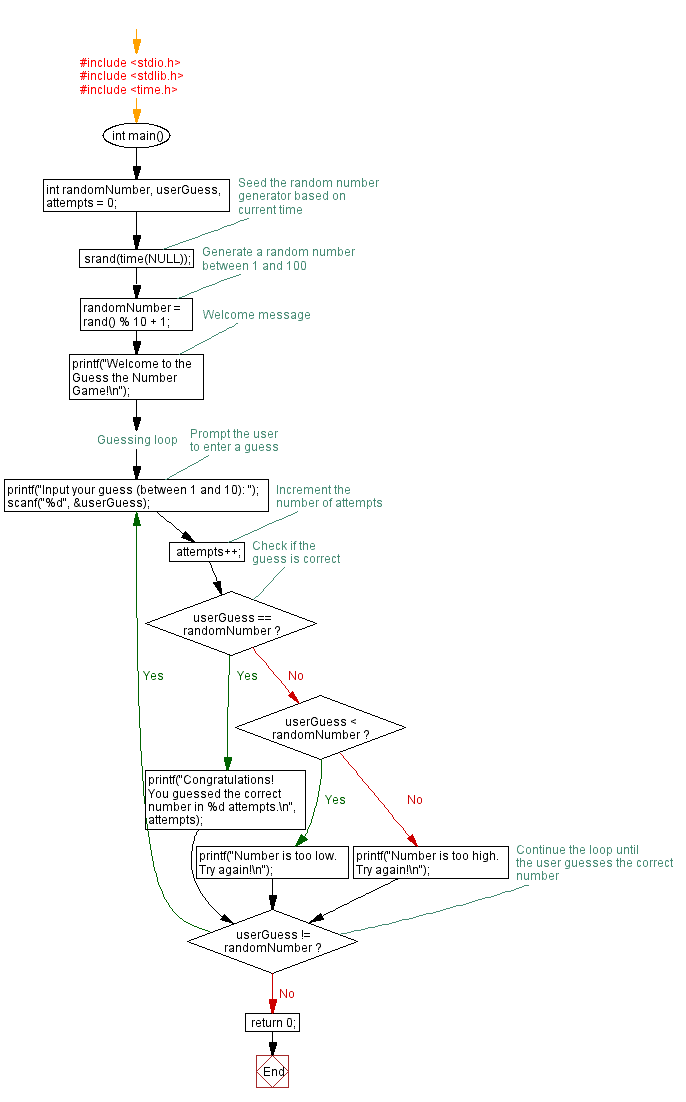
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate Sum of entered numbers with Do-While Loop.
Next: Calculate Sum of squares of digits with Do-While Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics