C language - File size
C File Handling : Exercise-19 with Solution
Write a program in C language to find the size of a given file.
Test Data:
Size of the said File: 34 bytes.
Sample Solution-1:
C Code:
#include <stdlib.h>
#include <stdio.h>
long getFileSize(const char *filename)
{
long result;
FILE *fh = fopen("i.txt", "rb");
fseek(fh, 0, SEEK_END);
result = ftell(fh);
fclose(fh);
return result;
}
int main(void)
{
printf("Size of the said File: %ld bytes.", getFileSize("input.txt"));
return 0;
}
Sample Output:
Size of the said File: 34 bytes
Flowchart:
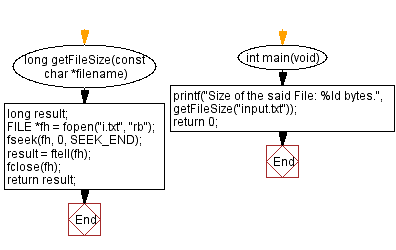
Sample Solution-2:
C Code:
#include <stdlib.h>
#include <stdio.h>
long getFileSize(const char *filename)
{
long result;
FILE *fh = fopen("i.txt", "rb");
fseek(fh, 0, SEEK_END);
result = ftell(fh);
fclose(fh);
return result;
}
int main(void)
{
printf("Size of the said File: %ld bytes.", getFileSize("input.txt"));
return 0;
}
Sample Output:
Size of the said File: 34 bytes
Note: This code run on Dev-C++ 5.11
Flowchart:
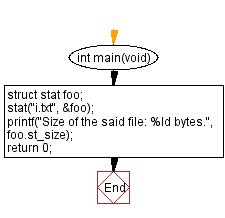
C Programming Code Editor:
Previous: File modification time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/file-handling/c-file-handling-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics