C Exercises: Read the file and count the number of lines
5. Count Lines in File
Write a program in C to find the number of lines in a text file.
Sample Solution:
C Code:
#include <stdio.h>
#define FSIZE 100
int main()
{
FILE *fptr;
int ctr = 0;
char fname[FSIZE];
char c;
printf("\n\n Read the file and count the number of lines :\n");
printf("--------------------------------------------------\n");
printf(" Input the file name to be opened : ");
scanf("%s",fname);
fptr = fopen(fname, "r");
if (fptr == NULL)
{
printf("Could not open file %s", fname);
return 0;
}
// Extract characters from file and store in character c
for (c = getc(fptr); c != EOF; c = getc(fptr))
if (c == '\n') // Increment count if this character is newline
ctr = ctr + 1;
fclose(fptr);
printf(" The lines in the file %s are : %d \n \n", fname, ctr-1);
return 0;
}
Sample Output:
Read the file and count the number of lines : -------------------------------------------------- Input the file name to be opened : test.txt The lines in the file test.txt are : 4
Flowchart:
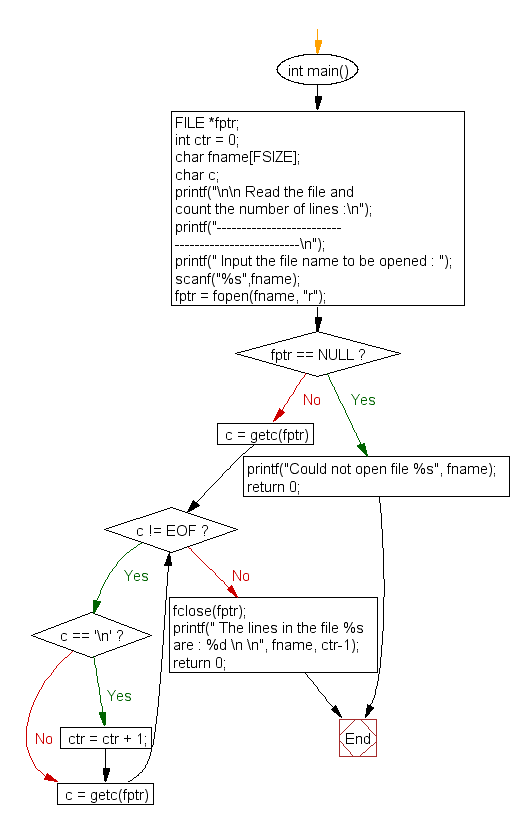
For more Practice: Solve these Related Problems:
- Write a C program to count the number of non-empty lines in a text file.
- Write a C program to count the number of lines in a file and also calculate the average line length.
- Write a C program to count the total number of lines in a file and then output the line count in a formatted report.
- Write a C program to count lines in a file while ignoring lines that start with a comment symbol (e.g., '#').
C Programming Code Editor:
Previous: Write a program in C to read the file and store the lines into an array.
Next: Write a program in C to find the content of the file and number of lines in a Text File.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.