C Exercises: Find the number and sum of all integer between 100 and 200, divisible by 9
39. Integers Divisible by 9 between 100 and 200
Write a program in C to find the number and sum of all integers between 100 and 200 which are divisible by 9.
The program should iterate through the numbers within the specified range, checking if each number is divisible by 9. If a number meets this condition, it should be included in the count, and its value should be added to the sum. Finally, the program will display the total count of such numbers and their sum.
Visual Presentation:
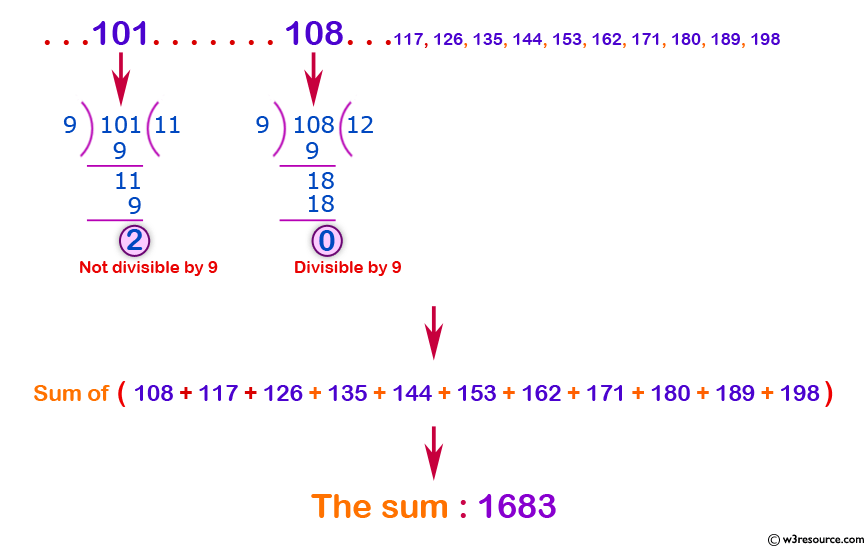
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, sum=0; // Declare variables for iteration and sum.
printf("Numbers between 100 and 200, divisible by 9 : \n"); // Print a message to indicate the output format.
for(i=101; i<200; i++) // Loop through numbers from 101 to 199.
{
if(i%9==0) // Check if the current number is divisible by 9.
{
printf("% 5d", i); // Print the number with formatting.
sum += i; // Add the divisible number to the sum.
}
}
printf("\n\nThe sum : %d \n", sum); // Print the total sum of divisible numbers.
}
Output:
Numbers between 100 and 200, divisible by 9 : 108 117 126 135 144 153 162 171 180 189 198 The sum : 1683
Flowchart:
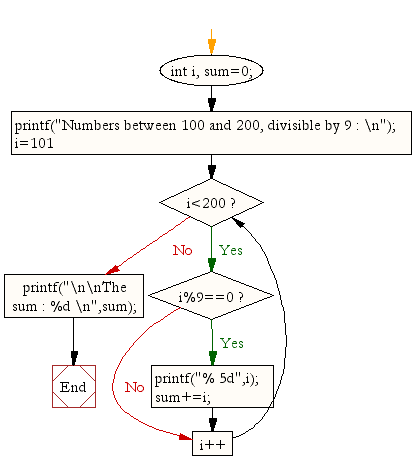
For more Practice: Solve these Related Problems:
- Write a C program to list all numbers between 100 and 200 divisible by 9 and then compute their product.
- Write a C program to find and sum all numbers between 100 and 200 divisible by 9 using recursion.
- Write a C program to display numbers divisible by 9 in the range 100–200 in reverse order and calculate their sum.
- Write a C program to list numbers divisible by 9 in a given range and then compute their average.
C Programming Code Editor:
Previous: Write a program in C to check whether a number is a palindrome or not.
Next: Write a C Program to display the pattern like pyramid using the alphabet.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.