C Exercises: Reverse a given string using an inline function
C inline function: Exercise-10 with Solution
Write a C program to reverse a given string using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
// inline function to reverse a given string
inline void reverse_string(char * str) {
int len = strlen(str);
for (int i = 0; i < len / 2; i++) {
char temp = str[i];
str[i] = str[len - i - 1];
str[len - i - 1] = temp;
}
}
int main() {
char str[100];
printf("Input a string: ");
fgets(str, 100, stdin);
str[strcspn(str, "\n")] = '\0'; // remove newline character from input
printf("Before reverse: %s\n", str);
reverse_string(str);
printf("After reverse: %s\n", str);
return 0;
}
Sample Output:
Input a string: Wikipedia Before reverse: Wikipedia After reverse: aidepikiW Input a string: C language Before reverse: C language After reverse: egaugnal C
Explanation:
In the above program, we define an inline function reverse_string() that takes a char pointer as input and reverses the string in its place. In the main() function, we get a string input from the user using the fgets() function. We remove the newline character from the input with strcspn() function. Use the printf() function to print the string before and after reversing, and return 0 to indicate the successful execution of the program.
Flowchart:
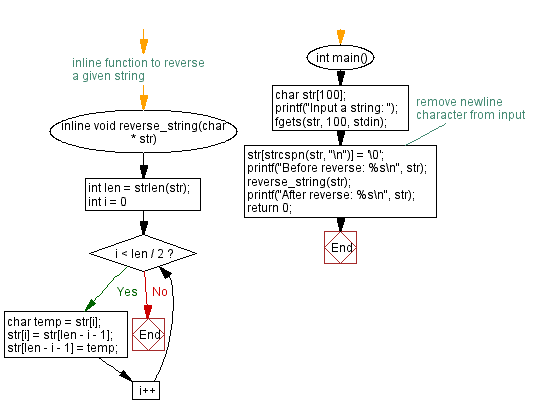
C Programming Code Editor:
Previous: Check if a given integer is even or odd using an inline function.
Next: Compute the sum of a variable number of integers passed as arguments using an inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics