C Exercises: Insert new node at the middle in a doubly linked list
6. Insert in the Middle of Doubly Linked List
Write a program in C to insert a new node in the middle of a doubly linked list.
Visual Presentation:
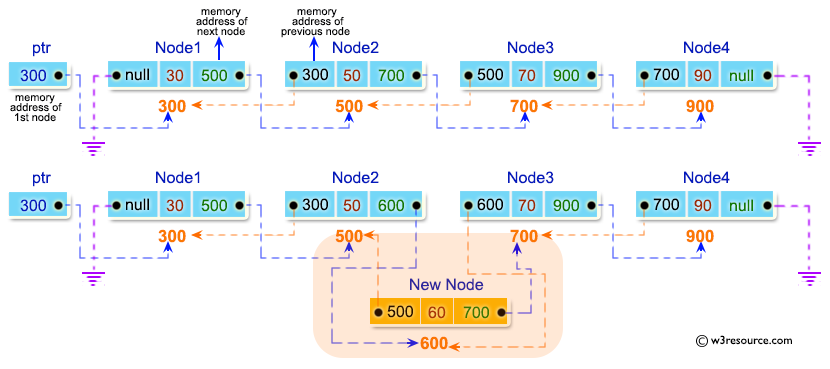
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
struct node {
int num;
struct node *preptr;
struct node *nextptr;
} *stnode, *ennode;
// Function prototypes
void DlListcreation(int n);
void DlLinsertNodeAtMiddle(int num, int pos);
void displayDlList(int a);
int main() {
int n, num1, a, insPlc;
stnode = NULL;
ennode = NULL;
// User input for the number of nodes
printf("\n\n Doubly Linked List : Insert new node at the middle in a doubly linked list :\n");
printf("----------------------------------------------------------------------------------\n");
printf(" Input the number of nodes (3 or more ): ");
scanf("%d", &n);
// Creating the doubly linked list
DlListcreation(n);
a = 1;
displayDlList(a);
// Inserting a node at a specified position
printf(" Input the position ( 2 to %d ) to insert a new node : ", n - 1);
scanf("%d", &insPlc);
// Checking if the position is valid
if (insPlc <= 1 || insPlc >= n) {
printf("\n Invalid position. Try again.\n ");
}
if (insPlc > 1 && insPlc < n) {
printf(" Input data for the position %d : ", insPlc);
scanf("%d", &num1);
DlLinsertNodeAtMiddle(num1, insPlc);
a = 2;
displayDlList(a);
}
return 0;
}
// Function to create a doubly linked list
void DlListcreation(int n) {
int i, num;
struct node *fnNode;
if (n >= 1) {
// Allocating memory for the first node
stnode = (struct node *)malloc(sizeof(struct node));
if (stnode != NULL) {
// Input data for the first node
printf(" Input data for node 1 : ");
scanf("%d", &num);
// Initializing values for the first node
stnode->num = num;
stnode->preptr = NULL;
stnode->nextptr = NULL;
ennode = stnode;
// Loop to create subsequent nodes and link them
for (i = 2; i <= n; i++) {
fnNode = (struct node *)malloc(sizeof(struct node));
if (fnNode != NULL) {
printf(" Input data for node %d : ", i);
scanf("%d", &num);
fnNode->num = num;
fnNode->preptr = ennode; // Linking new node with the previous node
fnNode->nextptr = NULL; // Setting next address of fnNode as NULL
ennode->nextptr = fnNode; // Linking previous node with the new node
ennode = fnNode; // Assigning new node as last node
} else {
printf(" Memory can not be allocated.");
break;
}
}
} else {
printf(" Memory can not be allocated.");
}
}
}
// Function to insert a node at a specified position in the doubly linked list
void DlLinsertNodeAtMiddle(int num, int pos) {
int i;
struct node *newnode, *tmp;
if (ennode == NULL) {
printf(" No data found in the list!\n");
} else {
tmp = stnode;
i = 1;
while (i < pos - 1 && tmp != NULL) {
tmp = tmp->nextptr;
i++;
}
if (tmp != NULL) {
newnode = (struct node *)malloc(sizeof(struct node));
newnode->num = num;
// Linking next address of new node with the next address of tmp node
newnode->nextptr = tmp->nextptr;
// Linking previous address of new node with the tmp node
newnode->preptr = tmp;
if (tmp->nextptr != NULL) {
tmp->nextptr->preptr = newnode; // (n+1)th node is linking with new node
}
tmp->nextptr = newnode; // (n-1)th node is linking with new node
} else {
printf(" The position you entered is invalid.\n");
}
}
}
// Function to display the doubly linked list
void displayDlList(int m) {
struct node *tmp;
int n = 1;
if (stnode == NULL) {
printf(" No data found in the List yet.");
} else {
tmp = stnode;
if (m == 1) {
printf("\n Data entered in the list are :\n");
} else {
printf("\n After insertion the new list are :\n");
}
// Loop to display nodes and their data
while (tmp != NULL) {
printf(" node %d : %d\n", n, tmp->num);
n++;
tmp = tmp->nextptr; // Moving the current pointer to the next node
}
}
}
Sample Output:
Doubly Linked List : Insert new node at the middle in a doubly linked list : ---------------------------------------------------------------------------------- Input the number of nodes (3 or more ): 3 Input data for node 1 : 2 Input data for node 2 : 4 Input data for node 3 : 5 Data entered in the list are : node 1 : 2 node 2 : 4 node 3 : 5 Input the position ( 2 to 2 ) to insert a new node : 2 Input data for the position 2 : 3 After insertion the new list are : node 1 : 2 node 2 : 3 node 3 : 4 node 4 : 5
Flowchart:
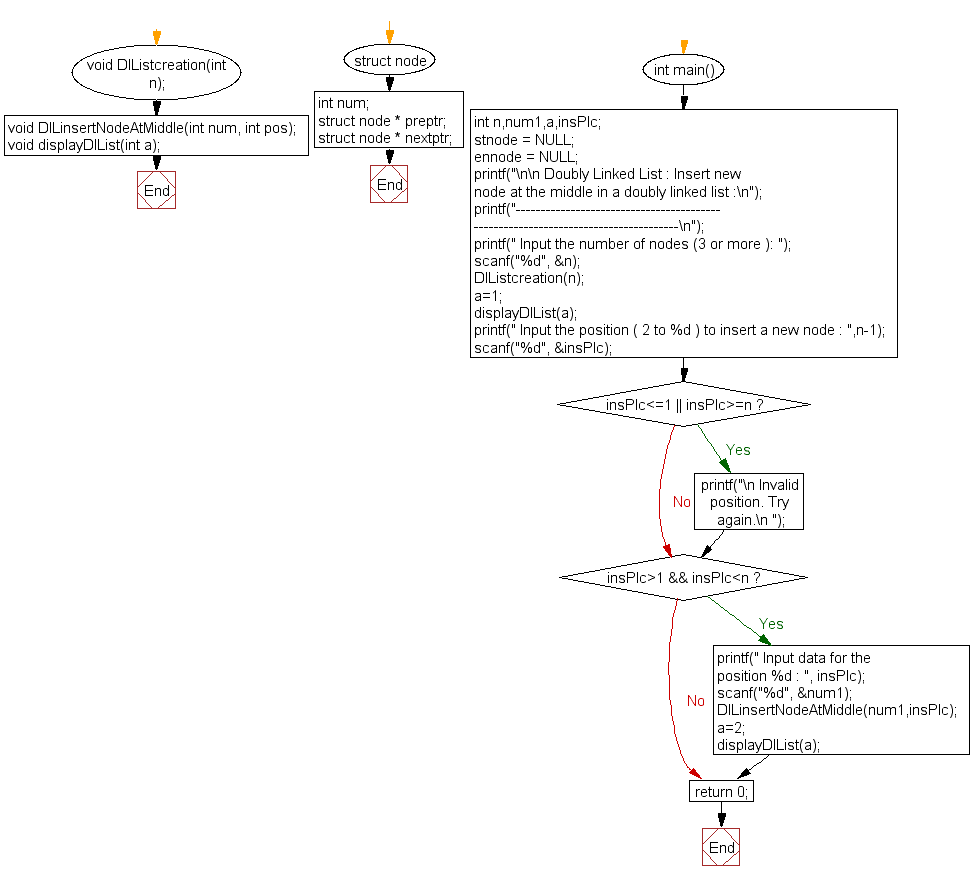
DlListcreation() :
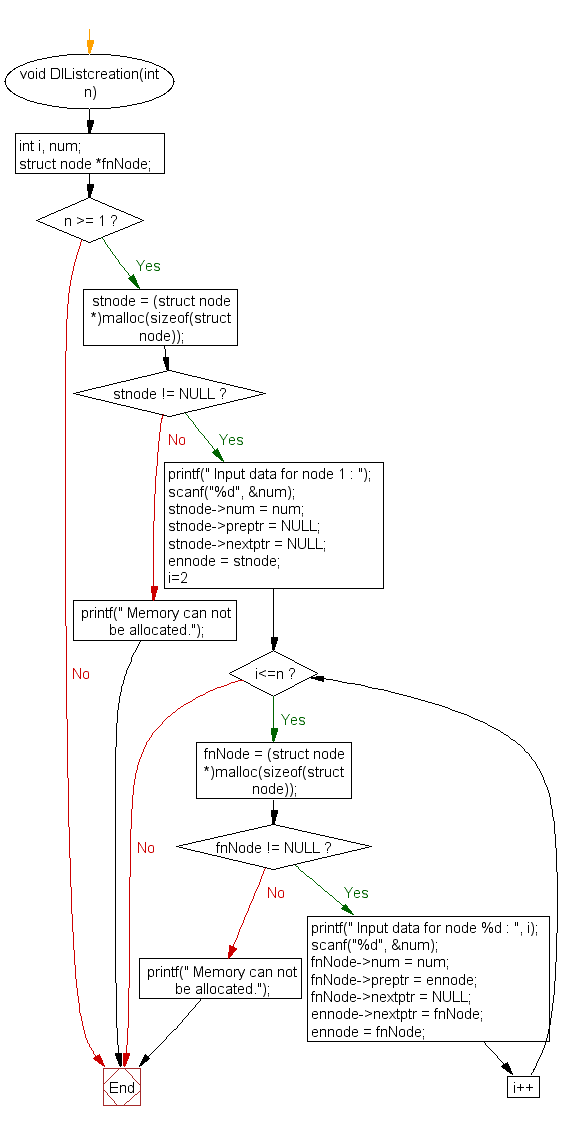
DlLinsertNodeAtMiddle() :
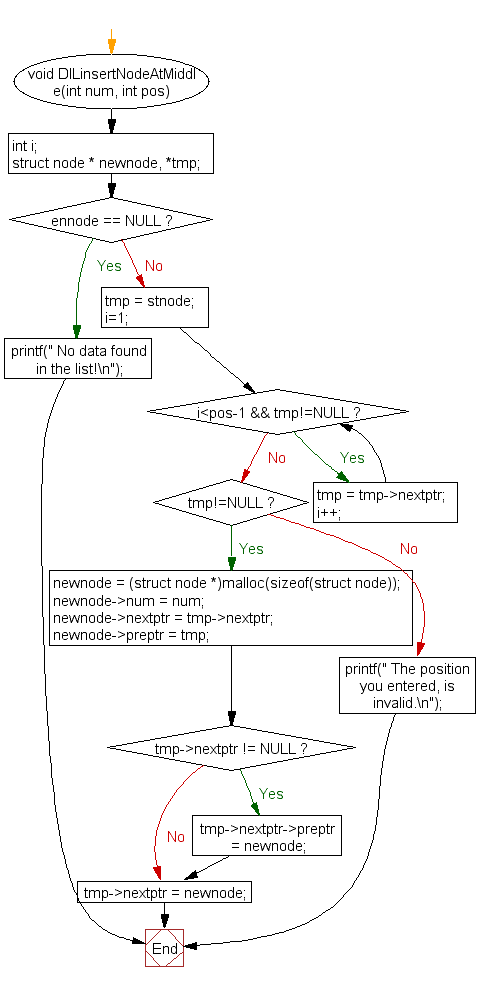
displayDlList() :
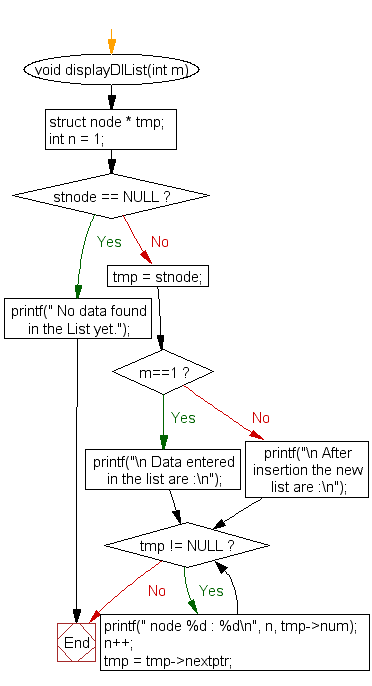
For more Practice: Solve these Related Problems:
- Write a C program to locate the middle of a doubly linked list and insert a new node right after the middle element.
- Write a C program to insert a node in the middle of a doubly linked list using the two-pointer (slow and fast) approach.
- Write a C program to insert a node at the center of a doubly linked list and then separately display the two halves.
- Write a C program to calculate the length of a doubly linked list first, then insert a node exactly at its midpoint.
C Programming Code Editor:
Previous: Write a program in C to insert a new node at any position in a doubly linked list.
Next: Write a program in C to delete a node from the beginning of a doubly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.