C Exercises: Delete node from any position of a doubly linked list
C Doubly Linked List : Exercise-9 with Solution
Write a program in C to delete a node from any position in a doubly linked list.
Visual Presentation:
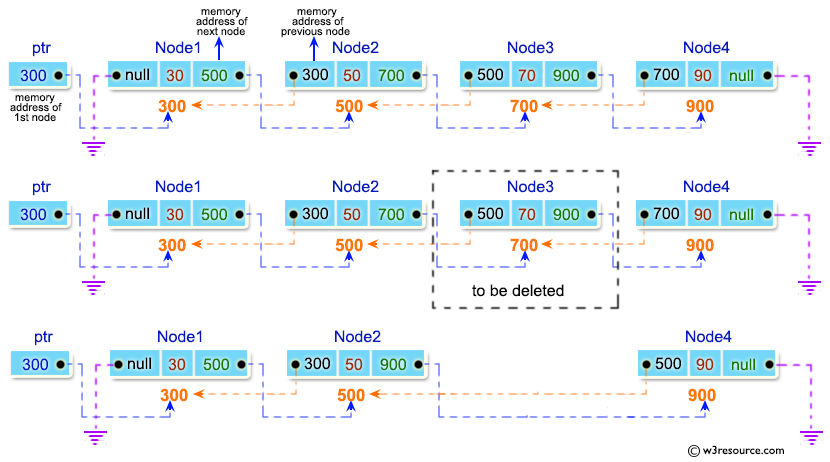
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
struct node {
int num;
struct node *preptr;
struct node *nextptr;
} *stnode, *ennode;
// Function prototypes
void DlListcreation(int n);
void DlListDeleteFirstNode();
void DlListDeleteLastNode();
void DlListDeleteAnyNode(int pos);
void displayDlList(int a);
int main() {
int n, a, insPlc;
stnode = NULL;
ennode = NULL;
// User input for the number of nodes
printf("\n\n Doubly Linked List : Delete node from any position of a doubly linked list :\n");
printf("----------------------------------------------------------------------------------\n");
printf(" Input the number of nodes (3 or more ): ");
scanf("%d", &n);
// Creating the doubly linked list
DlListcreation(n);
a = 1;
displayDlList(a);
// Asking for the position to delete a node
printf(" Input the position ( 1 to %d ) to delete a node : ", n);
scanf("%d", &insPlc);
// Checking for valid position and deleting the node
if (insPlc < 1 || insPlc > n) {
printf("\n Invalid position. Try again.\n ");
} else if (insPlc >= 1 && insPlc <= n) {
DlListDeleteAnyNode(insPlc);
a = 2;
displayDlList(a);
}
return 0;
}
// Function to create a doubly linked list
void DlListcreation(int n) {
int i, num;
struct node *fnNode;
if (n >= 1) {
// Allocating memory for the first node
stnode = (struct node *)malloc(sizeof(struct node));
if (stnode != NULL) {
// Input data for the first node
printf(" Input data for node 1 : ");
scanf("%d", &num);
stnode->num = num;
stnode->preptr = NULL;
stnode->nextptr = NULL;
ennode = stnode;
// Loop to create subsequent nodes and link them
for (i = 2; i <= n; i++) {
fnNode = (struct node *)malloc(sizeof(struct node));
if (fnNode != NULL) {
printf(" Input data for node %d : ", i);
scanf("%d", &num);
fnNode->num = num;
fnNode->preptr = ennode; // Linking new node with the previous node
fnNode->nextptr = NULL; // Setting next address of fnNode as NULL
ennode->nextptr = fnNode; // Linking previous node with the new node
ennode = fnNode; // Assigning new node as last node
} else {
printf(" Memory can not be allocated.");
break;
}
}
} else {
printf(" Memory can not be allocated.");
}
}
}
// Function to delete a node from any position in the doubly linked list
void DlListDeleteAnyNode(int pos) {
struct node *curNode;
int i;
curNode = stnode;
for (i = 1; i < pos && curNode != NULL; i++) {
curNode = curNode->nextptr;
}
if (pos == 1) {
DlListDeleteFirstNode();
} else if (curNode == ennode) {
DlListDeleteLastNode();
} else if (curNode != NULL) {
curNode->preptr->nextptr = curNode->nextptr;
curNode->nextptr->preptr = curNode->preptr;
free(curNode); // Delete the n node
} else {
printf(" The given position is invalid!\n");
}
}
// Function to delete the first node from the doubly linked list
void DlListDeleteFirstNode() {
struct node *NodeToDel;
if (stnode == NULL) {
printf(" Delete is not possible. No data in the list.\n");
} else {
NodeToDel = stnode;
stnode = stnode->nextptr; // Move the next address of starting node to 2 node
stnode->preptr = NULL; // Set previous address of starting node to NULL
free(NodeToDel); // Delete the first node from memory
}
}
// Function to delete the last node from the doubly linked list
void DlListDeleteLastNode() {
struct node *NodeToDel;
if (ennode == NULL) {
printf(" Delete is not possible. No data in the list.\n");
} else {
NodeToDel = ennode;
ennode = ennode->preptr; // Move the previous address of the last node to 2nd last node
ennode->nextptr = NULL; // Set the next address of last node to NULL
free(NodeToDel); // Delete the last node
}
}
// Function to display the doubly linked list
void displayDlList(int m) {
struct node *tmp;
int n = 1;
if (stnode == NULL) {
printf(" No data found in the List yet.");
} else {
tmp = stnode;
if (m == 1) {
printf("\n Data entered in the list are :\n");
} else {
printf("\n After deletion the new list are :\n");
}
// Loop to display nodes and their data
while (tmp != NULL) {
printf(" node %d : %d\n", n, tmp->num);
n++;
tmp = tmp->nextptr; // Moving the current pointer to the next node
}
}
}
Sample Output:
Doubly Linked List : Delete node from any position of a doubly linked list : ---------------------------------------------------------------------------------- Input the number of nodes (3 or more ): 3 Input data for node 1 : 1 Input data for node 2 : 2 Input data for node 3 : 3 Data entered in the list are : node 1 : 1 node 2 : 2 node 3 : 3 Input the position ( 1 to 3 ) to delete a node : 3 After deletion the new list are : node 1 : 1 node 2 : 2
Flowchart:
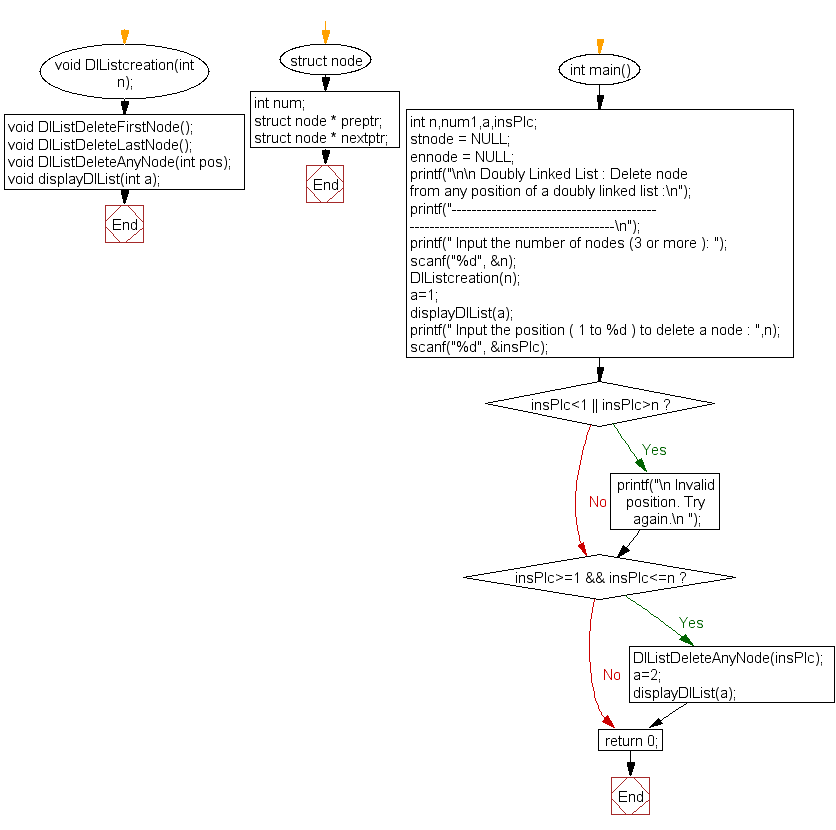
DlListcreation() :
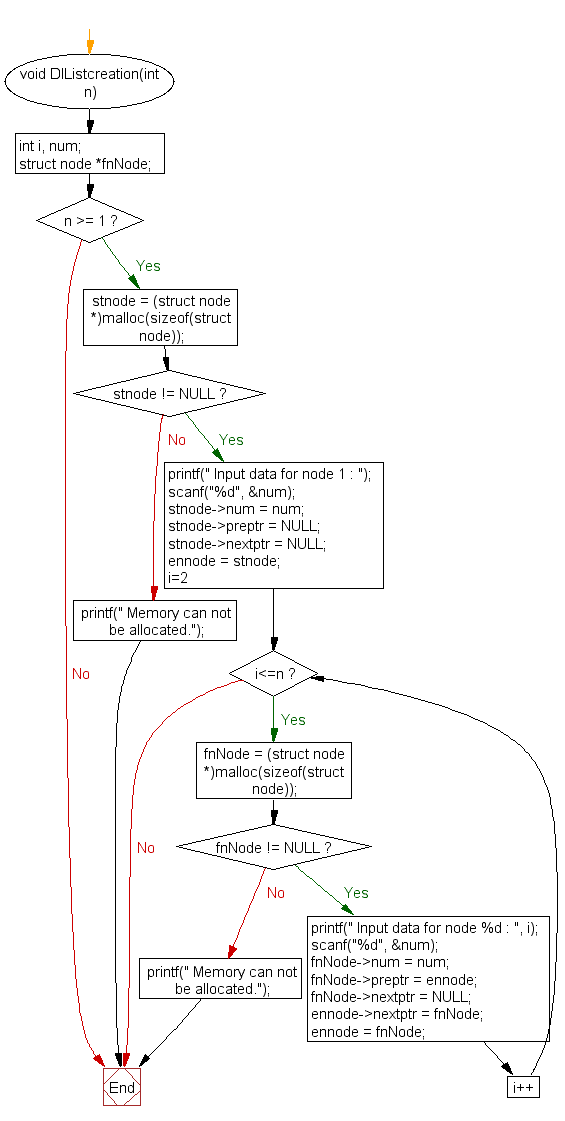
DlListDeleteAnyNode() :
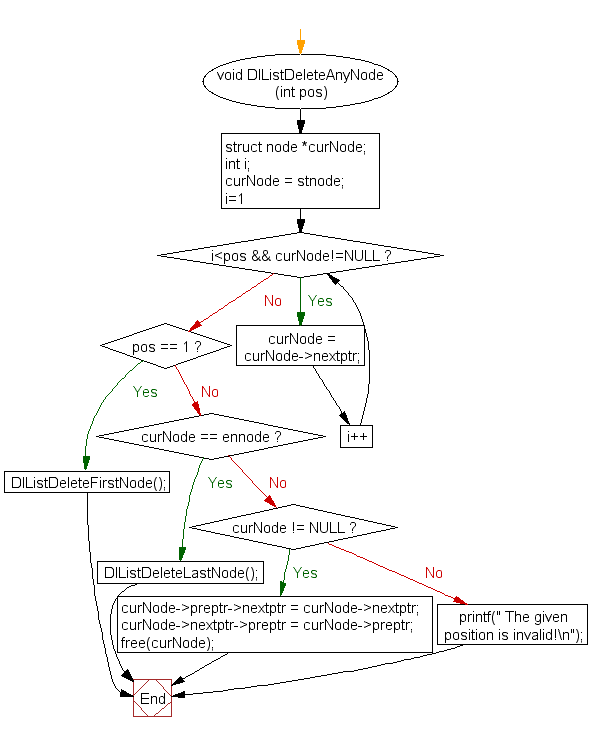
DlListDeleteFirstNode() :
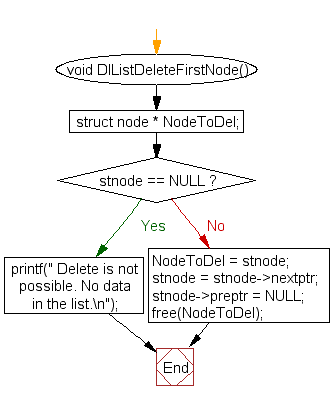
DlListDeleteLastNode() :
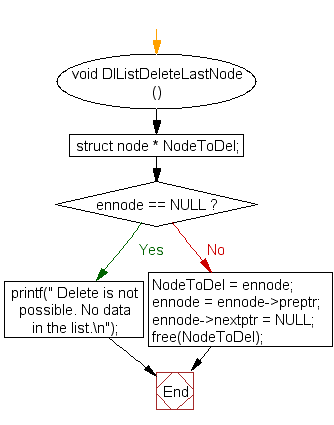
displayDlList() :
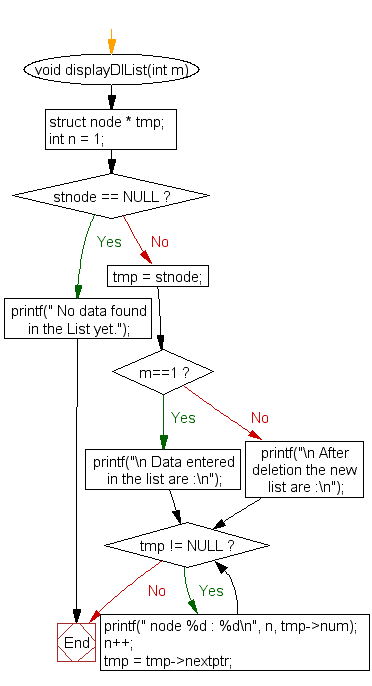
C Programming Code Editor:
Previous: Write a program in C to delete a node from the last of a doubly linked list.
Next: Write a program in C to delete a node from middle of a doubly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/linked_list/c-linked_list-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics