C Exercises: Check whether an integer is a palindrome or not
2. Palindrome Integer Check Variants
Write a C program to check whether an integer is a palindrome or not. An integer is a palindrome when it reads the same forward as backward.
Example:
Input:
i = 1221
i = -121
i = 100
Output:
Is Palindrome: 1
Is Palindrome: 0
Is Palindrome: 0
Visual Presentation:
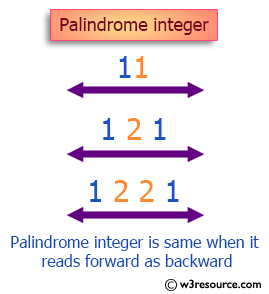
Sample Solution:
C Code:
#include <stdio.h>
#include <stdbool.h>
// Function to check if a number is a palindrome
bool is_Palindrome(int i) {
int n, d, y = 0;
// Check if the number is negative; negative numbers cannot be palindromes
if (i < 0)
return false;
n = i;
// Reverse the number and compare it with the original number
while (n) {
d = n % 10;
// Check for potential overflow
if (y > (0x7fffffff - d) / 10)
return false; // Return false if overflow is detected
y = y * 10 + d; // Reverse the number
n = n / 10; // Move to the next digit
}
return (y == i); // Return true if the reversed number is equal to the original number
}
int main(void)
{
int i = 1221;
printf("Original integer: %d ", i);
printf("\nIs Palindrome: %d ", is_Palindrome(i));
i = -121;
printf("\nOriginal integer: %d ", i);
printf("\nIs Palindrome: %d ", is_Palindrome(i));
i = 100;
printf("\nOriginal integer: %d ", i);
printf("\nIs Palindrome: %d ", is_Palindrome(i));
return 0;
}
Sample Output:
Original integer: 1221 Is Palindrome: 1 Original integer: -121 Is Palindrome: 0 Original integer: 100 Is Palindrome: 0
Flowchart:
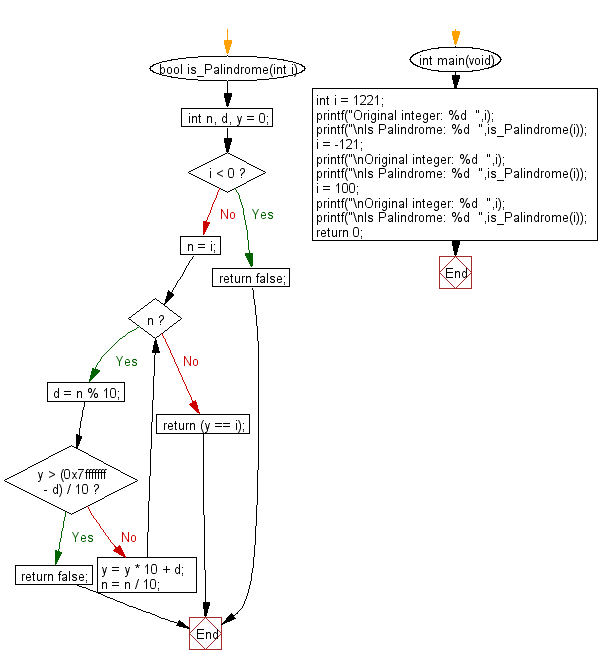
For more Practice: Solve these Related Problems:
- Write a C program to determine if an integer is a palindrome by comparing its digit array without converting to a string.
- Write a C program to check palindromic property of an integer using arithmetic operations only.
- Write a C program to test palindrome property while ignoring the sign and any trailing zeros.
- Write a C program to verify the palindrome property of an integer by reversing half of its digits and comparing.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to reverse the digits of a given integer
Next: Write a C program to divide two integers (dividend and divisor) without using multiplication, division and mod operator.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.