C Exercises: Find Disarium numbers between 1 to 1000
16. Disarium Numbers Between 1 and 1000 Variants
Write a program in C to find Disarium numbers between 1 and 1000.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to count the number of digits in a given number
int DigiCount(int n)
{
int ctr_digi = 0;
int tmpx = n;
// Count the number of digits
while (tmpx)
{
tmpx = tmpx / 10;
ctr_digi++;
}
return ctr_digi; // Return the count of digits
}
// Function to check if a number is a Disarium Number
bool chkDisarum(int n)
{
int ctr_digi = DigiCount(n); // Get the count of digits in the number
int s = 0;
int x = n;
int pr;
// Calculate the sum of powers of digits
while (x)
{
pr = x % 10;
s = s + pow(pr, ctr_digi--); // Add the power of the digit to the sum
x = x / 10; // Move to the next digit
}
return (s == n); // Return true if the sum equals the original number
}
// Main function
int main()
{
int i;
printf("\n\n Find Disarium Numbers between 1 to 1000: \n");
printf(" ---------------------------------------------\n");
printf(" The Disarium numbers are: \n");
// Check for Disarium Numbers in the range of 1 to 1000
for (i = 1; i <= 1000; i++)
{
if (chkDisarum(i)) // If i is a Disarium Number
printf("%d ", i); // Print the Disarium Number
}
printf("\n");
return 0;
}
Sample Output:
The Disarium numbers are: 1 2 3 4 5 6 7 8 9 89 135 175 518 598
Visual Presentation:
Flowchart:
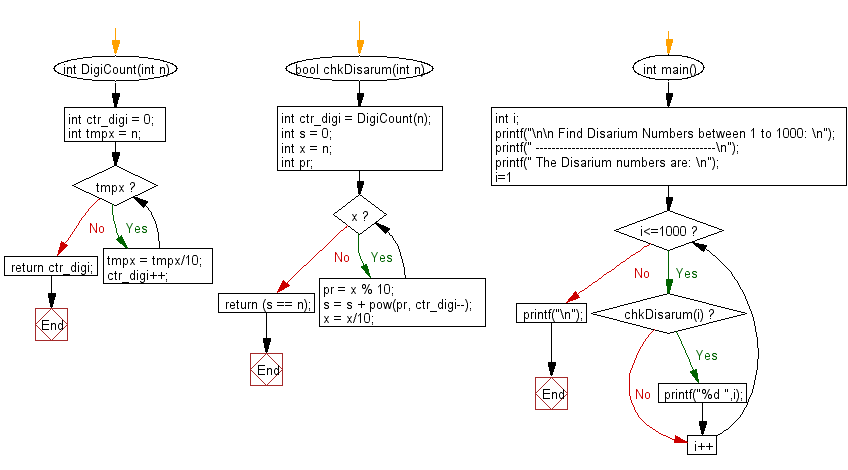
-->
For more Practice: Solve these Related Problems:
- Write a C program to generate disarium numbers within user-defined lower and upper limits.
- Write a C program to count and list disarium numbers along with their powered digit sums.
- Write a C program to display each disarium number with a breakdown of its digit contributions.
- Write a C program to optimize disarium number detection using memoization for power calculations.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a number is Disarium or not.
Next: Write a program in C to check if a number is Harshad Number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.