C Exercises: Check whether a number is a Duck Number or not
C Numbers: Exercise-23 with Solution
Write a program in C to check whether a number is a Duck Number or not.
Test DataInput a number: 3210
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
int main()
{
int dno, dkno, r, flg; // Variable declaration: dno stores the original number, dkno stores the number for manipulation, r stores the remainder, flg is a flag for checking
flg = 0; // Initializing flag to 0
// Printing information about the program and asking for user input
printf("\n\n Check whether a number is a Duck Number or not: \n");
printf(" ----------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &dkno); // Reading input from the user
dno = dkno; // Storing the original number
while (dkno > 0) // Loop to check if the number is a Duck Number
{
if (dkno % 10 == 0) // Checking if any digit of the number is zero
{
flg = 1; // Setting flag to 1 if zero is found
break; // Exiting the loop if zero is found
}
dkno /= 10; // Moving to the next digit in the number
}
if (dno > 0 && flg == 1) // Checking if the original number is positive and a zero was found
{
printf(" The given number is a Duck Number.\n"); // Printing if the number is a Duck Number
}
else
{
printf(" The given number is not a Duck Number.\n"); // Printing if the number is not a Duck Number
}
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input a number: 3210 The given number is a Duck Number.
Visual Presentation:
Flowchart:
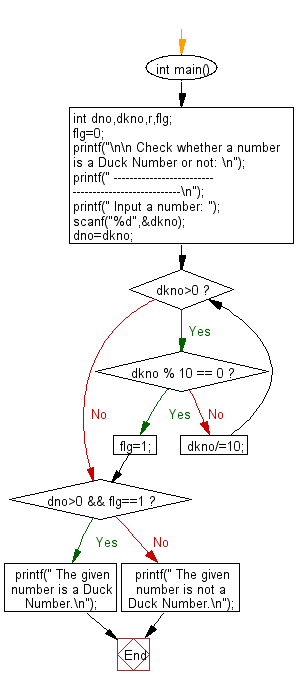
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in c++ to find the the Authomorphic numbers between 1 to 1000.
Next: Write a program in C to find Duck Numbers between 1 to 500.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/numbers/c-numbers-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics