C Exercises: Display first 10 Fermat numbers
30. Fermat Numbers Variants
Write a program in C to display the first 10 Fermat numbers.
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <math.h>
int main()
{
int n = 0; // Variable 'n' initialized to 0 to track the iteration count
double result; // Variable to store the calculated Fermat number
printf("\n\n Display first 10 Fermat numbers:\n");
printf("-------------------------------------\n");
printf(" The first 10 Fermat numbers are: \n");
// Loop to calculate and display the first 10 Fermat numbers
while (n <= 10)
{
result = pow(2, pow(2, n)) + 1; // Calculating the nth Fermat number using the formula 2^(2^n) + 1
n++; // Incrementing 'n' for the next iteration
printf("%lf \n", result); // Printing the calculated Fermat number
}
}
Sample Output:
The first 10 Fermat numbers are: 3.000000 5.000000 17.000000 257.000000 65537.000000 4294967297.000000 18446744073709551616.000000 340282366920938463463374607431768211456.000000 115792089237316195423570985008687907853269984665640564039457584007913129639936.000000 13407807929942597099574024998205846127479365820592393377723561443721764030073546976801874298166903427690031858 186486050853753882811946569946433649006084096.000000 inf
Visual Presentation:
Flowchart:
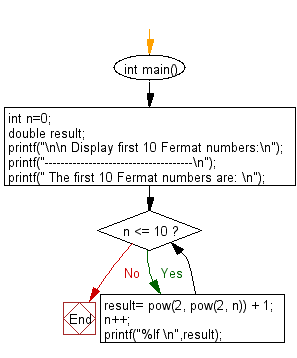
For more Practice: Solve these Related Problems:
- Write a C program to generate Fermat numbers using iterative exponentiation.
- Write a C program to verify Fermat numbers with large integer arithmetic libraries.
- Write a C program to compute Fermat numbers and check the primality of each number.
- Write a C program to display Fermat numbers in scientific notation for extremely large outputs.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a given number is a perfect cube or not.
Next: Write a program in C to find any number between 1 and n that can be expressed as the sum of two cubes in two (or more) different ways.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.