C Exercises: Check if a number is Mersenne number or not
32. Mersenne Number Check Variants
Write a program in C to check if a number is a Mersenne number or not.
Test DataInput a number: 127
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int n, p, ans, i, n1; // Variables for input number, powers of 2, result, loop control, and incremented number 'n1'
double result; // Variable to store the result of the power operation
printf("\n\n Check whether a given number is a Mersenne number or not:\n");
printf("------------------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &n); // Reading the input number from the user
n1 = n + 1; // Incrementing the input number by 1
p = 0; // Initializing the variable 'p' for powers of 2
ans = 0; // Initializing the result variable 'ans' to check if the number is a Mersenne number
// Loop to find if 'n1' is a power of 2
for (i = 0;; i++)
{
p = (int)pow(2, i); // Calculating powers of 2 using the 'pow' function
if (p > n1)
{
break; // Exiting the loop if the power exceeds 'n1'
}
else if (p == n1)
{
printf(" %d is a Mersenne number.\n", n); // Printing if 'n1' is a Mersenne number
ans = 1; // Setting the result variable to indicate 'n' is a Mersenne number
}
}
if (ans == 0)
{
printf(" %d is not a Mersenne number.\n", n); // Printing if 'n' is not a Mersenne number
}
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input a number: 127 127 is a Mersenne number.
Visual Presentation:
Flowchart:
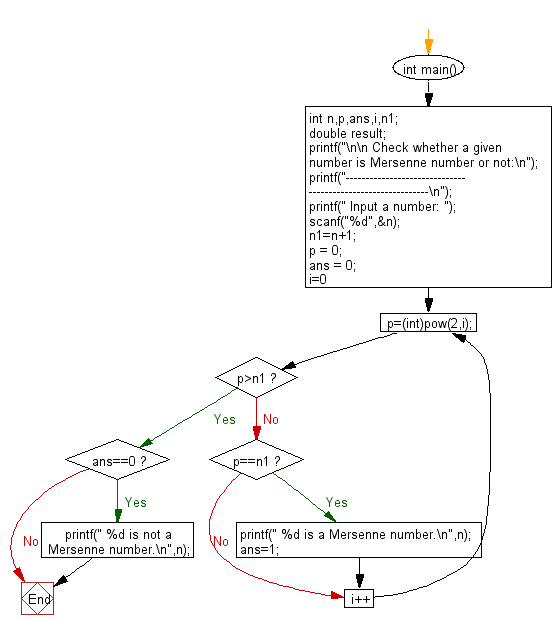
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is a Mersenne number by verifying it has the form 2^p - 1.
- Write a C program to test Mersenne numbers using bit manipulation techniques.
- Write a C program to verify Mersenne numbers and output the corresponding exponent p.
- Write a C program to optimize Mersenne number checking using logarithmic functions.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find any number between 1 and n that can be expressed as the sum of two cubes in two (or more) different ways.
Next: Write a program in C to generate mersenne primes within a range of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.