C Exercises: Find the factorial of a given number
C Pointer : Exercise-12 with Solution
Write a program in C to find the factorial of a given number using pointers.
Visual Presentation:
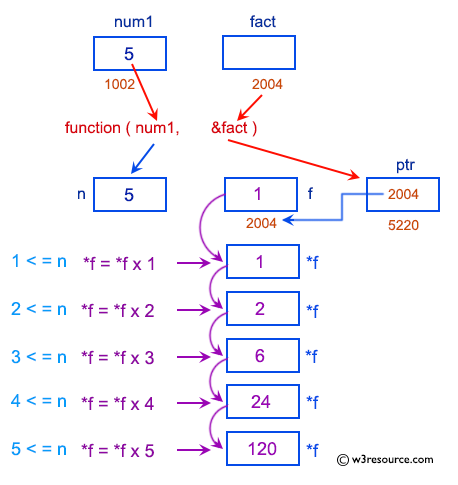
Sample Solution:
C Code:
#include <stdio.h>
// Function prototype to find factorial of a number using pointers
void findFact(int, int*);
int main() {
int fact; // Variable to store factorial
int num1; // Variable to store user input
printf("\n\n Pointer : Find the factorial of a given number :\n");
printf("------------------------------------------------------\n");
// Input a number from the user
printf(" Input a number : ");
scanf("%d", &num1);
// Call the function to calculate factorial passing the address of 'fact'
findFact(num1, &fact);
// Display the factorial of the given number
printf(" The Factorial of %d is : %d \n\n", num1, fact);
return 0;
}
// Function definition to find factorial using pointers
void findFact(int n, int *f) {
int i;
*f = 1; // Initialize the factorial to 1
// Loop to calculate factorial of the given number
for (i = 1; i <= n; i++) {
*f = *f * i; // Calculate factorial by multiplying *f with i
}
}
Sample Output:
Pointer : Find the factorial of a given number : ------------------------------------------------------ Input a number : 5 The Factorial of 5 is : 120
Flowchart:
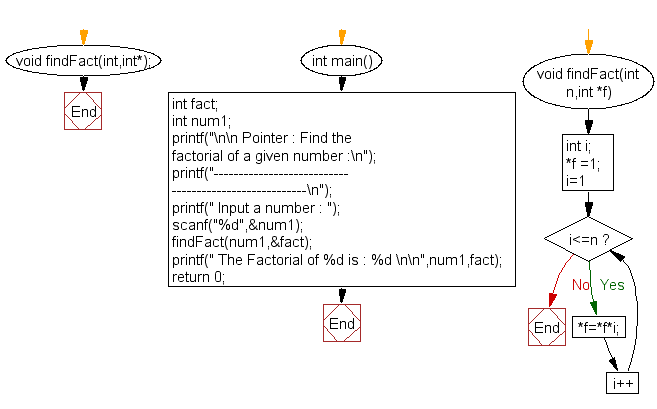
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to swap elements using call by reference.
Next: Write a program in C to count the number of vowels and consonants in a string using a pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics