C Exercises: Check if a given string is valid or not
C Programming Challenges: Exercise-10 with Solution
Write a C program to check if a given string is valid or not. The string contains the characters '(', ')', '{', '}', '[' and ']'. The string is valid if the open brackets must be closed with the same type of bracket and in the correct order.
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
static bool is_valid_Parentheses(char *s)
{
int n = 0, cap = 100;
char *tstack = malloc(cap);
while (*s != '\0') {
switch(*s) {
case '(':
case '[':
case '{':
if (n + 1 >= cap) {
cap *= 2;
tstack = realloc(tstack, cap);
}
tstack[n++] = *s;
break;
case ')':
if (tstack[--n] != '(') return false;
break;
case ']':
if (tstack[--n] != '[') return false;
break;
case '}':
if (tstack[--n] != '{') return false;
break;
default:
return false;
}
s++;
}
return n == 0;
}
int main(void)
{
//char **vbracket = "()[]{}";
char **vbracket = "([)]";
printf("%s\n", is_valid_Parentheses(vbracket) ? "true" : "false");
return 0;
}
Sample Output:
false
Flowchart:
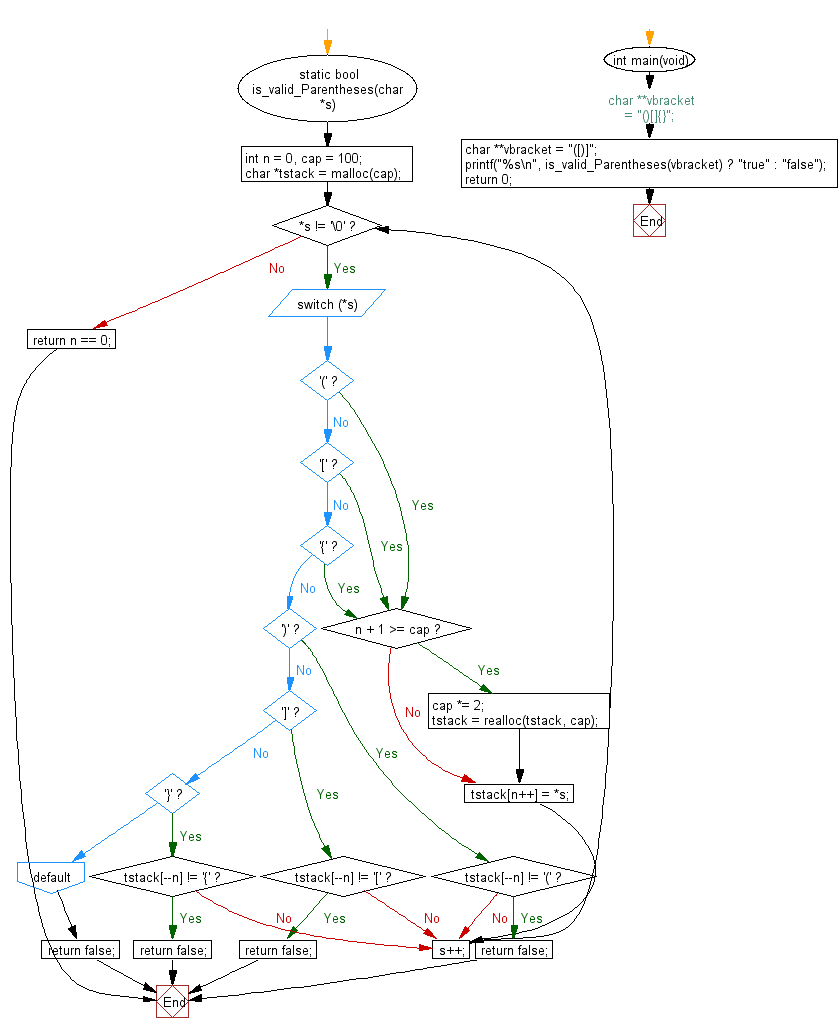
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: All quadruplets in an array with zero sum.
Next C Programming Exercise: Combinations of parentheses from n pairs.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/practice/c-programming-practice-exercises-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics