C Exercises: First missing +ve integer in unsorted array
28. Descending Prime Numbers (Strictly Decreasing Digits) Variants
Write a C program to find the first missing positive integer in a given unsorted integer array.
C Code:
//https://github.com/begeekmyfriend/leetcode/blob/master/041_first_missing_positive/missing_positive.c
#include <stdio.h>
#include <stdlib.h>
static inline void swap_val(int *x, int *y)
{
int tmp = *x;
*x = *y;
*y = tmp;
}
static int first_Missing_Positive_num(int* nums, int nums_size)
{
if (nums_size < 1) {
return 1;
}
int i = 0;
while (i < nums_size) {
if (nums[i] != i + 1 && nums[i] > 0 && nums[i] <= nums_size && nums[i] != nums[nums[i] - 1]) {
swap_val(nums + i, nums + nums[i] - 1);
} else {
i++;
}
}
for (i = 0; i < nums_size; i++) {
if (nums[i] != i + 1) break;
}
return i + 1;
}
int main()
{
int i, count = 4;
int nums[4] = {3,4,-1,1};
printf("%d\n", first_Missing_Positive_num(nums, count));
return 0;
}
Sample Output:
2
Flowchart:
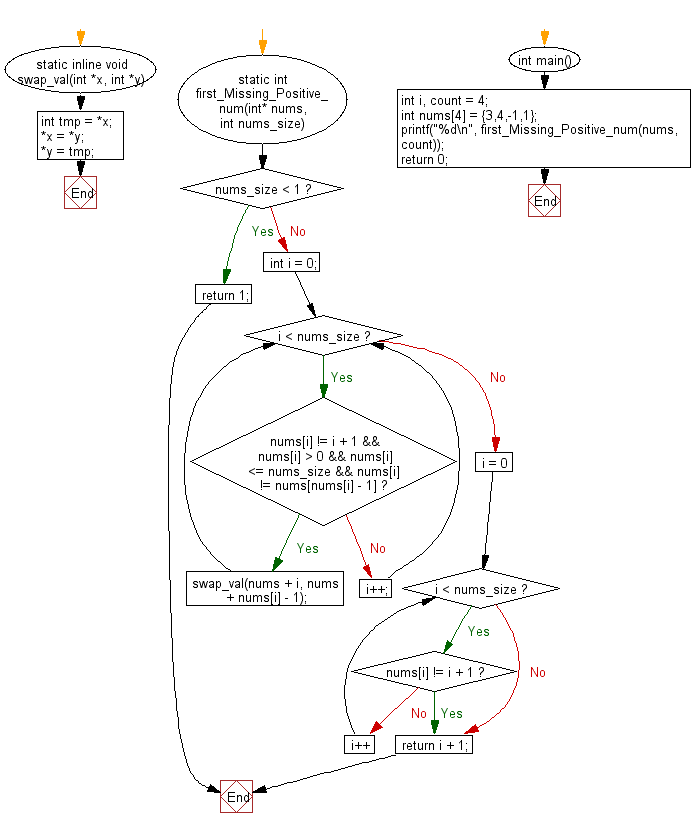
For more Practice: Solve these Related Problems:
- Write a C program to generate all prime numbers with digits in strictly descending order.
- Write a C program to count and list primes that have strictly decreasing digits from a given range.
- Write a C program to display descending prime numbers and compare their distribution with ascending ones.
- Write a C program to compute the average of prime numbers with strictly descending digits.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Product of four adjacent numbers in a 20x20 grid.
Next C Programming Exercise: Minimum number of characters to make a palindrome.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.