C Exercises: Arithmetic and geometric progression series
31. Progression Type & Next Member Variants
Write a C program to find the type of the progression (arithmetic progression/geometric progression) and the next successive member of a given three successive members of a sequence.
According to Wikipedia, an arithmetic progression (AP) is a sequence of numbers such that the difference of any two successive members of the sequence is a constant. For instance, the sequence 3, 5, 7, 9, 11, 13,... is an arithmetic progression with common difference 2. For this problem, we will limit ourselves to arithmetic progression whose common difference is a non-zero integer. On the other hand, a geometric progression (GP) is a sequence of numbers where each term after the first is found by multiplying the previous one by a fixed non-zero number called the common ratio. For example, the sequence 2, 6, 18, 54,... is a geometric progression with common ratio 3. For this problem, we will limit ourselves to geometric progression whose common ratio is a non-zero integer.
C Code:
#include<stdio.h>
int main()
{
float n;
float arr[3];
printf("Input three successive numbers of the sequence:\n");
scanf("%llf%llf%llf",&arr[0],&arr[1],&arr[2]);
if ((arr[0]== 0) && (arr[1]== 0) && (arr[2]== 0))
{
printf("Wrong Numbers");
return 0;
}
if (((arr[1]/arr[0])!=(arr[2]/arr[1])) && ((arr[1]-arr[0])!=(arr[2]-arr[1])))
{
printf("Not a AP/GP Sequence");
return 0;
}
if ((arr[1]-arr[0])==(arr[2]-arr[1]))
{
n = 2*(arr[2])-arr[1];
printf("\nAP sequence, Next number of the sequence: %f", n);
}
else
{
n=(arr[2]*arr[2])/arr[1];
printf("\nGP sequence, Next number of the sequence: %f", n);
}
return 0;
}
Sample Output:
Input three successive numbers of the sequence: 2 6 18 54 GP sequence, Next number of the sequence: 54.000000
Flowchart:
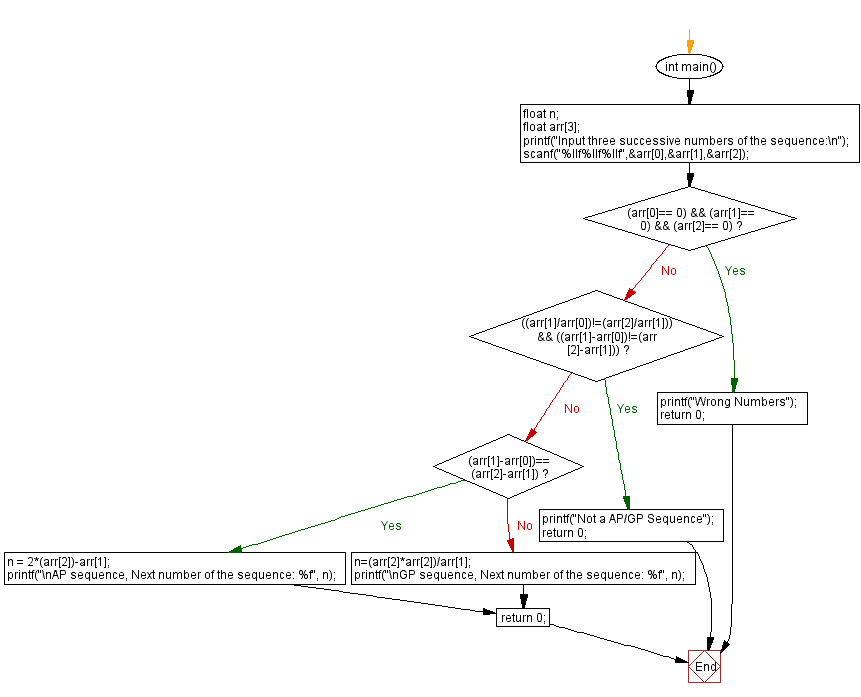
For more Practice: Solve these Related Problems:
- Write a C program to determine if three numbers form an arithmetic progression and predict the next term.
- Write a C program to check if three numbers form a geometric progression and calculate the succeeding member.
- Write a C program to analyze a triplet of numbers to determine if they form any progression and output the type and next value.
- Write a C program to validate a sequence as either arithmetic or geometric and then compute the next term based on user choice.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Prime number in strictly ascending decimal digit order.
Next C Programming Exercise: Prime number in strictly descending decimal digit order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.