C Exercises: Convert a given roman number to an integer
7. Roman Numeral to Integer Variants
Write a C program to convert a Roman number to an integer.
Roman numerals are represented by seven different symbols: I, V, X, L, C, D and M. Symbol Value I 1 V 5 X 10 L 50 C 100 D 500 M 1000
C Code:
#include <stdio.h>
#include <stdlib.h>
static int roman_to_integer(char c)
{
switch(c) {
case 'I':
return 1;
case 'V':
return 5;
case 'X':
return 10;
case 'L':
return 50;
case 'C':
return 100;
case 'D':
return 500;
case 'M':
return 1000;
default:
return 0;
}
}
int roman_to_int (char *s)
{
int i, int_num = roman_to_integer(s[0]);
for (i = 1; s[i] != '\0'; i++) {
int prev_num = roman_to_integer(s[i - 1]);
int cur_num = roman_to_integer(s[i]);
if (prev_num < cur_num) {
int_num = int_num - prev_num + (cur_num - prev_num);
} else {
int_num += cur_num;
}
}
return int_num;
}
int main(void)
{
char *str1 = "XIV";
printf("Original Roman number: %s", str1);
printf("\nRoman to integer: %d", roman_to_int(str1));
return 0;
}
Sample Output:
Original Roman number: XIV Roman to integer: 14
Pictorial Presentation:
Flowchart:
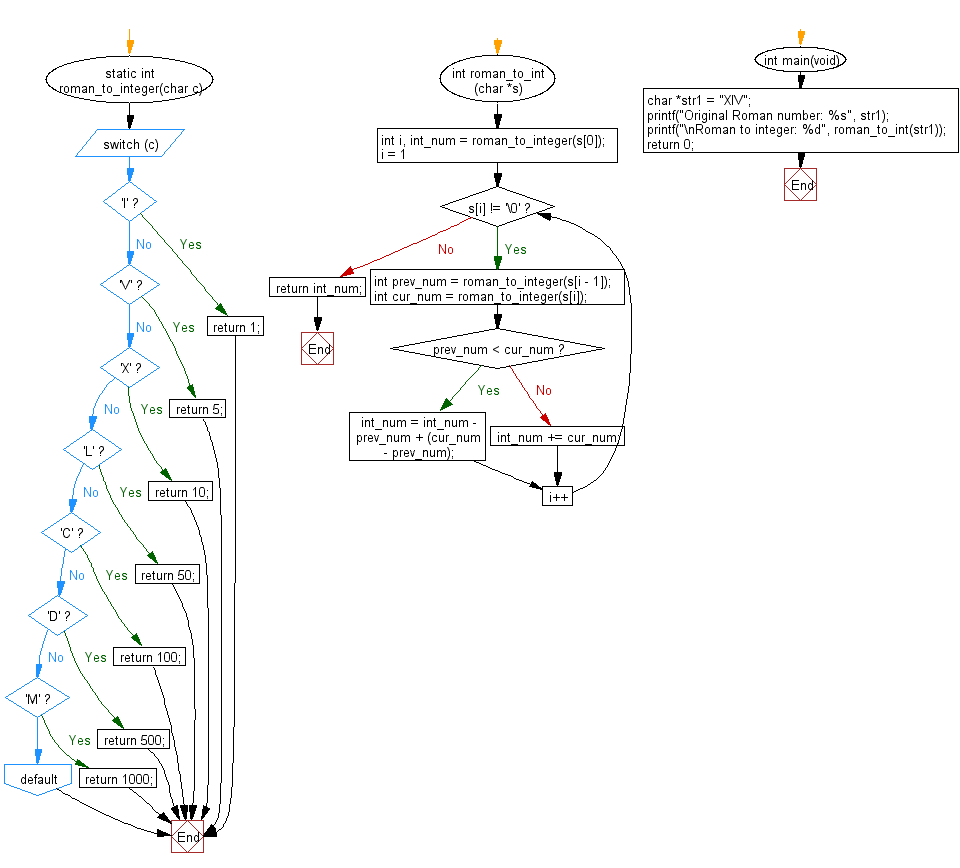
For more Practice: Solve these Related Problems:
- Write a C program to convert a Roman numeral string to an integer, handling subtractive cases correctly.
- Write a C program to convert multiple Roman numeral strings to integers and store the results in an array.
- Write a C program to implement a case-insensitive Roman numeral to integer conversion.
- Write a C program to validate the input Roman numeral and return an error for invalid sequences.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Convert a given integer to roman number.
Next C Programming Exercise: Sum of unique triplets in a set is 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.