C Exercises: Check a given string is Palindrome or not
16. Palindrome String Recursion Variants
Write a C program to check whether a given string is a palindrome or not using recursion.
Pictorial Presentation:
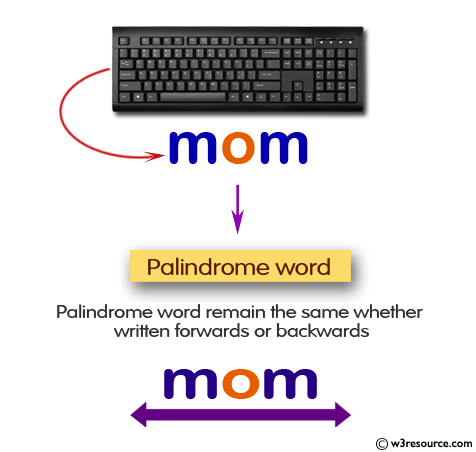
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
void checkPalindrome(char [], int);
int main()
{
char wordPal[25];
printf("\n\n Recursion : Check a given string is Palindrome or not :\n");
printf("----------------------------------------------------------\n");
printf(" Input a word to check for palindrome : ");
scanf("%s", wordPal);
checkPalindrome(wordPal, 0);//call the function for checking Palindorem
return 0;
}
void checkPalindrome(char wordPal[], int index)
{
int len = strlen(wordPal) - (index + 1);
if (wordPal[index] == wordPal[len])
{
if (index + 1 == len || index == len)
{
printf(" The entered word is a palindrome.\n\n");
return;
}
checkPalindrome(wordPal, index + 1);//calling the function itself recursively
}
else
{
printf(" The entered word is not a palindrome.\n\n");
}
}
Sample Output:
Recursion : Check a given string is Palindrome or not : ---------------------------------------------------------- Input a word to check for palindrome : mom The entered word is a palindrome.
Explanation:
void checkPalindrome(char wordPal[], int index) { int len = strlen(wordPal) - (index + 1); if (wordPal[index] == wordPal[len]) { if (index + 1 == len || index == len) { printf(" The entered word is a palindrome.\n\n"); return; } checkPalindrome(wordPal, index + 1);//calling the function itself recursively } else { printf(" The entered word is not a palindrome.\n\n"); } }
This function ‘checkPalindrome()’ takes a string ‘wordPal’ and an integer ‘index’ as parameters. It checks if the given string is a palindrome or not by comparing the first and last characters of the string, and then recursively checks the remaining characters in the same way.
If the first and last characters match, the function calls itself with the updated index to check the remaining characters. If they do not match, the function returns "The entered word is not a palindrome." as output.
Time complexity and space complexity:
The time complexity of this function is O(n) since it traverses the string once, where n is the length of the string.
The space complexity of this function is O(n) because of the recursive calls, which create new stack frames each time, and the maximum number of stack frames that can be created is equal to the length of the string.
Flowchart:
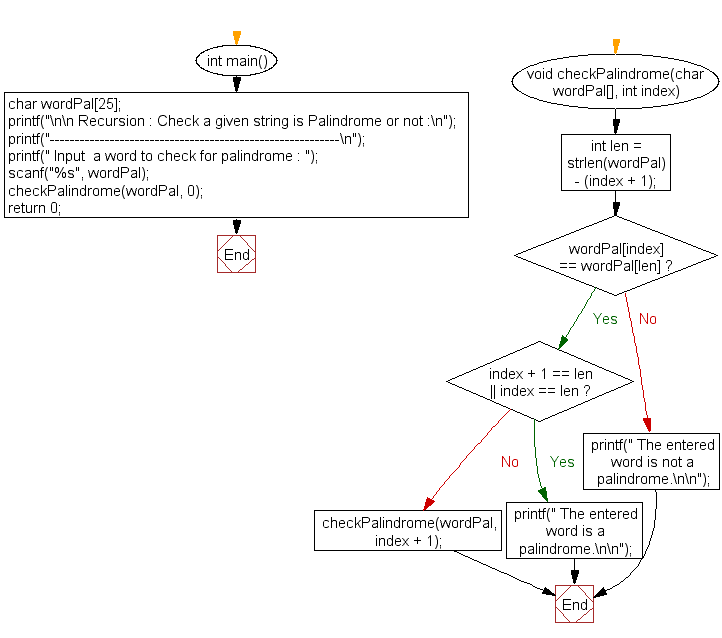
For more Practice: Solve these Related Problems:
- Write a C program to check if a string is a palindrome ignoring case recursively.
- Write a C program to verify if a sentence (with spaces) is a palindrome using recursion.
- Write a C program to return the longest palindromic substring from a string recursively.
- Write a C program to check if a string is a mirrored palindrome (same when reversed and rotated) using recursion.
C Programming Code Editor:
Previous: Write a program in C to multiply two matrix using recursion.
Next: Write a program in C to calculate the power of any number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.