C Exercises: Copy One string to another
C Recursion : Exercise-19 with Solution
Write a program in C to copy one string to another using recursion.
Pictorial Presentation:
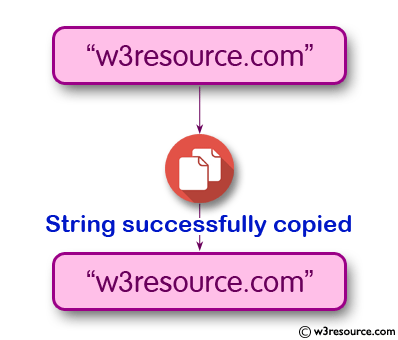
Sample Solution:
C Code:
#include <stdio.h>
void copyString(char [], char [], int);
int main()
{
char stng1[20], stng2[20];
printf("\n\n Recursion : Copy One string to another :\n");
printf("---------------------------------------------\n");
printf(" Input the string to copy : ");
scanf("%s", stng1);
copyString(stng1, stng2, 0);
printf("\n The string successfully copied.\n\n");
printf(" The first string is : %s\n", stng1);
printf(" The copied string is : %s\n\n", stng2);
return 0;
}
void copyString(char stng1[], char stng2[], int ctr)
{
stng2[ctr] = stng1[ctr];
if (stng1[ctr] == '\0')
return;
copyString(stng1, stng2, ctr + 1);
}
Sample Output:
Recursion : Copy One string to another : --------------------------------------------- Input the string to copy : w3resource The string successfully copied. The first string is : w3resource The copied string is : w3resource
Explanation:
void copyString(char stng1[], char stng2[], int ctr) { stng2[ctr] = stng1[ctr]; if (stng1[ctr] == '\0') return; copyString(stng1, stng2, ctr + 1); }
The copyString() function takes two character arrays stng1 and stng2 and an integer ctr as arguments. It recursively copies the characters of stng1 to stng2 starting from index ctr until it finds a null character (\0) in stng1. At that point, it returns from the function.
- The character at index ctr of stng1 is copied to the corresponding index of stng2.
- If the character at index ctr of stng1 is null (\0), the function returns.
- Otherwise, the function calls itself with ctr+1 as the new index.
- The function continues to copy the characters until it reaches the end of stng1.
Time complexity and space complexity:
The time complexity of this function is O(n) where n is the length of the input string stng1.
The space complexity is O(n) as well since the function uses recursion to copy the string, which creates a new stack frame for each recursive call.
Flowchart:
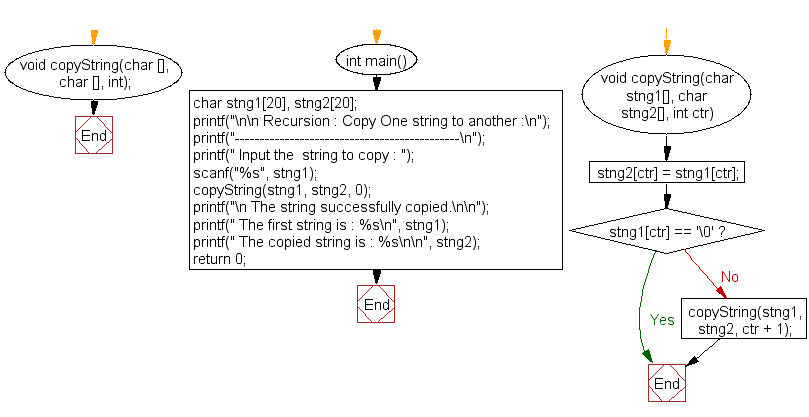
C Programming Code Editor:
Previous: Write a program in C to find the Hailstone Sequence of a given number upto 1.
Next: Write a program in C to find the first capital letter in a string using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/recursion/c-recursion-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics