C Exercises: Get reverse of a string
9. Reverse String Recursion Variants
Write a program in C to reverse a string using recursion.
Pictorial Presentation:
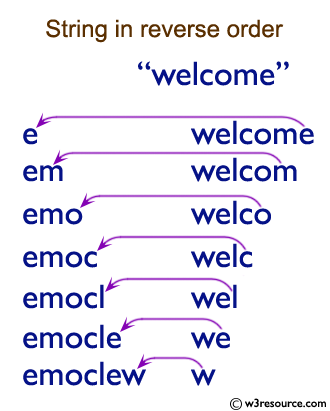
Sample Solution:
C Code:
#include<stdio.h>
#define MAX 100
char* ReverseOfString(char[]);
int main()
{
char str1[MAX],*revstr;
printf("\n\n Recursion : Get reverse of a string :\n");
printf("------------------------------------------\n");
printf(" Input any string: ");
scanf("%s",str1);
revstr = ReverseOfString(str1);//call the function ReverseOfString
printf(" The reversed string is: %s\n\n",revstr);
return 0;
}
char* ReverseOfString(char str1[])
{
static int i=0;
static char revstr[MAX];
if(*str1)
{
ReverseOfString(str1+1);//calling the function ReverseOfString itself
revstr[i++] = *str1;
}
return revstr;
}
Sample Output:
Recursion : Get reverse of a string : ------------------------------------------ Input any string: w3resource The reversed string is: ecruoser3w
Explanation:
char* ReverseOfString(char str1[]) { static int i=0; static char revstr[MAX]; if(*str1) { ReverseOfString(str1+1);//calling the function ReverseOfString itself revstr[i++] = *str1; } return revstr; }
This function 'ReverseOfString ()' takes a string as input. The function starts by declaring two static variables, i and revstr. The variable i is used to keep track of the index of the reversed string and is initialized to 0. The variable revstr is used to store the reversed string.
The function checks if the first character of the input string is not null. If it is not null, the function calls itself recursively with the input string pointer incremented by 1 (i.e., the address of the second character). This recursively moves through the string until it reaches the end.
For each recursive call, the function stores the current character at the i-th index of the ‘revstr ‘ array and increments the i variable. Finally, when the first character is null, the function returns the revstr array which contains the reversed string.
Time complexity and space complexity:
The time complexity of this function is O(n), where n is the length of the input string, since it recursively iterates through the entire string.
The space complexity is also O(n), as the size of the ‘revstr’ array is proportional to the length of the input string.
Flowchart:
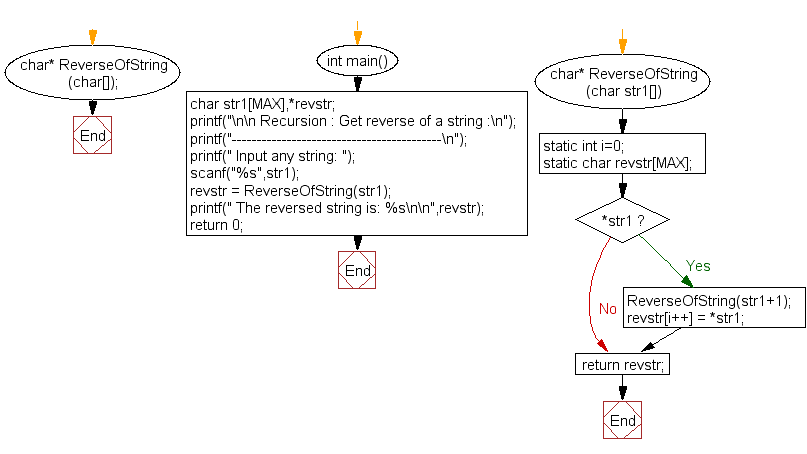
For more Practice: Solve these Related Problems:
- Write a C program to reverse a string word by word using recursion.
- Write a C program to reverse only the vowels in a string using recursion.
- Write a C program to check if a string equals its reverse by using recursion.
- Write a C program to reverse a string in place using recursion and pointer arithmetic.
C Programming Code Editor:
Previous: Write a program in C to get the largest element of an array using recursion.
Next: Write a program in C to find the Factorial of a number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.