C Exercises: Counting sort algorithm
8. Counting Sort Variants
Write a C program for counting sort.
Note:
According to Wikipedia "In computer science, counting sort is an algorithm for sorting a collection of objects according to keys that are small integers; that is, it is an integer sorting algorithm. It operates by counting the number of objects that have each distinct key value, and using arithmetic on those counts to determine the positions of each key value in the output sequence. Its running time is linear in the number of items and the difference between the maximum and minimum key values, so it is only suitable for direct use in situations where the variation in keys is not significantly greater than the number of items. However, it is often used as a subroutine in another sorting algorithm, radix sort, that can handle larger keys more efficiently".
Sample Solution:
Sample C Code:
#include <stdio.h>
/* Counting sort function */
int counting_sort(int a[], int k, int n) {
int i, j;
int b[15], c[100];
// Initialize the count array
for (i = 0; i <= k; i++)
c[i] = 0;
// Count the occurrences of each element in the input array
for (j = 1; j <= n; j++)
c[a[j]] = c[a[j]] + 1;
// Update the count array to contain the cumulative count of elements
for (i = 1; i <= k; i++)
c[i] = c[i] + c[i - 1];
// Build the sorted array based on the count array
for (j = n; j >= 1; j--) {
b[c[a[j]]] = a[j];
c[a[j]] = c[a[j]] - 1;
}
// Display the sorted array
printf("The Sorted array is: ");
for (i = 1; i <= n; i++)
printf("%d,", b[i]);
}
int main() {
int n, k = 0, a[15], i;
// Input the number of elements
printf("Input number of elements: ");
scanf("%d", &n);
// Input the array elements one by one
printf("Input the array elements one by one: \n");
for (i = 1; i <= n; i++) {
scanf("%d", &a[i]);
// Update the maximum element value
if (a[i] > k) {
k = a[i];
}
}
// Call the counting_sort function to sort the array
counting_sort(a, k, n);
printf("\n");
return 0;
}
Sample Input:
3 12 15 56
Sample Output:
Input number of elements: Input the array elements one by one: The Sorted array is : 12,15,56,
Flowchart:
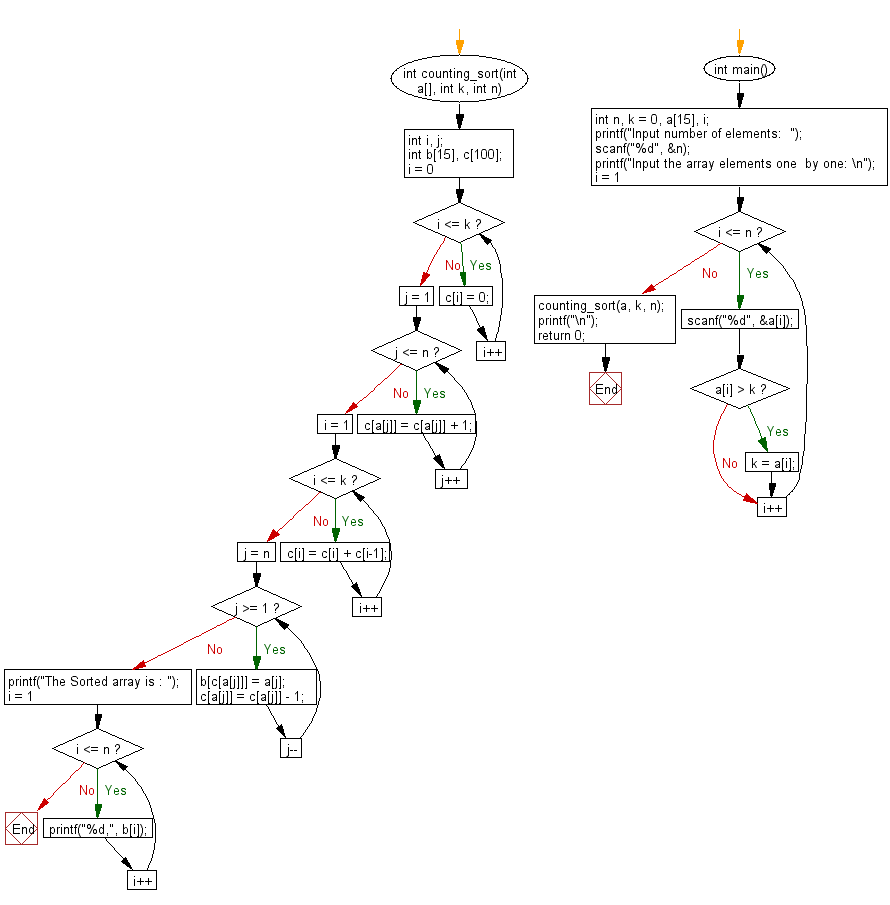
For more Practice: Solve these Related Problems:
- Write a C program to implement counting sort on an array of integers and verify its stability with duplicate values.
- Write a C program to use counting sort to sort the characters in a string and output the sorted string.
- Write a C program to implement counting sort on an array with a large range and optimize memory usage.
- Write a C program to count element frequencies in an array using counting sort and reconstruct the sorted array.
C Programming Code Editor:
Previous: Write a C program to sort a list of elements using the Radix sort algorithm.
Next: Write a C program to display sorted list using Gnome Sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.