C Exercises: Set a function that will be executed on termination of a program
C Variable Type : Exercise-15 with Solution
Write a C program to set a function that will be executed on termination of a program.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
// Function to be executed when the program exits.
void newFunctionOne (void)
{
puts (" Here is the message returning from newFunctionOne.");
}
// Another function to be executed when the program exits.
void newFunctionTwo (void)
{
puts (" Here is the message returning from newFunctionTwo.");
}
int main () // Start of the main function.
{
atexit (newFunctionOne); // Register 'newFunctionOne' to be executed at program exit.
atexit (newFunctionTwo); // Register 'newFunctionTwo' to be executed at program exit.
puts ("\n This is the message from main function."); // Print a message from the main function.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
This is the message from main function. Here is the message returning from newFunctionTwo. Here is the message returning from newFunctionOne
Flowchart:
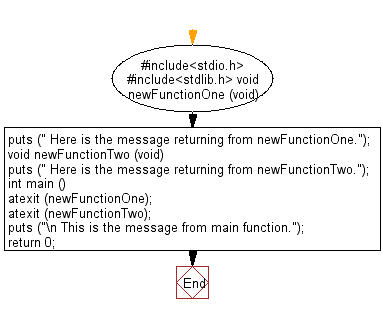
Previous: Write a C program to convert a string to a double.
Next: Write a C program to return the absolute value of an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/variable-type/c-variable-type-exercises-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics