C++ Exercises: Find the element that appears once in an array of integers and every other element appears twice
23. Find Unique Element Where Others Appear Twice
Write a C++ program to find the element that appears once in an array of integers and every other element appears twice.
Visual Presentation:
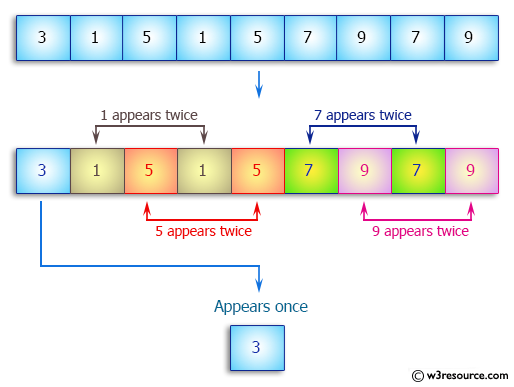
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to find the single element in an array with duplicate elements
int search_single_element(int array1[], int s1)
{
int result = array1[0]; // Initialize the result with the first element of the array
// Iterate through the array starting from the second element
for (int i = 1; i < s1; i++)
result = result ^ array1[i]; // Perform bitwise XOR operation to find the single element
return result; // Return the single element found
}
// Main function
int main()
{
int array1[] = {3, 1, 5, 1, 5, 7, 9, 7, 9}; // Declaration and initialization of the array
int se; // Variable to store the single element
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of the array
cout << "Original array: "; // Output label for the original array
// Output each element of the original array
for (int i = 0; i < s1; i++)
cout << array1[i] << " ";
se = search_single_element(array1, s1); // Call the function to find the single element
cout << "\nSingle element: " << se; // Output the single element
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 3 1 5 1 5 7 9 7 9 Single element: 3
Flowchart:
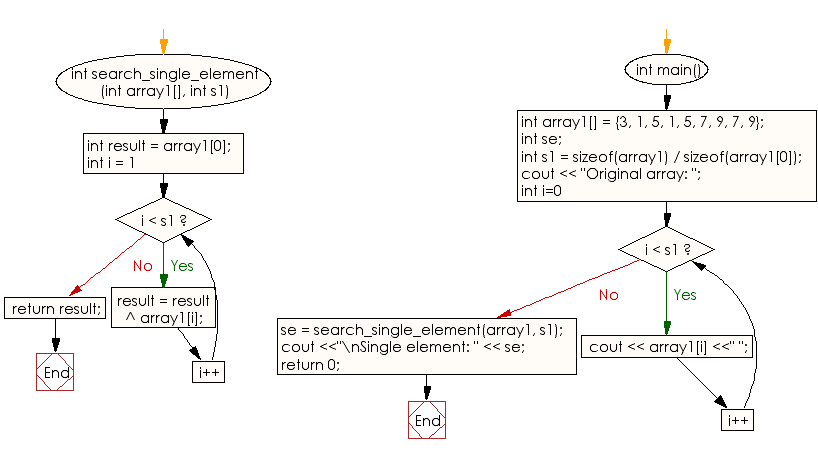
For more Practice: Solve these Related Problems:
- Write a C++ program to identify the single element in an array that does not have a duplicate, using bitwise XOR.
- Write a C++ program that reads an array and outputs the element that appears only once while every other element appears twice.
- Write a C++ program to scan through an array using XOR to isolate the unique element.
- Write a C++ program that employs a loop to find the non-repeating element in an array where all other elements are paired.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the missing element from two given arrays of integers except one element.
Next: Write a C++ program to find the first repeating element in an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.