C++ Exercises: Compute the sum of the two given integer values
Triple Sum for Same Values
Write a C++ program to compute the sum of two given integer values. If the two values are the same, then return triple their sum.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to calculate a result based on the equality of two integers x and y
int test(int x, int y)
{
return x == y ? (x + y) * 3 : x + y; // If x equals y, return the sum of x and y multiplied by 3. Otherwise, return the sum of x and y.
}
// Main function
int main()
{
cout << test(1, 2) << endl; // Output the result of test function with arguments 1 and 2
cout << test(3, 2) << endl; // Output the result of test function with arguments 3 and 2
cout << test(2, 2) << endl; // Output the result of test function with arguments 2 and 2
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
3 5 12
Visual Presentation:
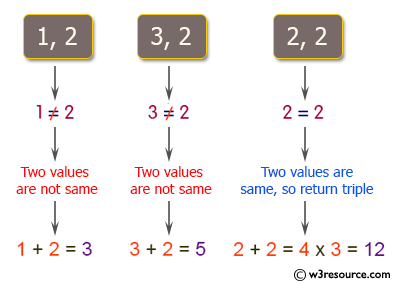
Flowchart:
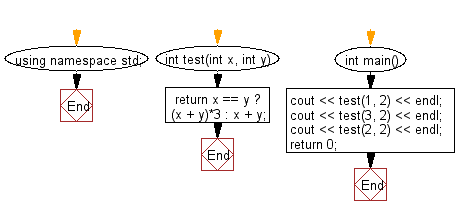
For more Practice: Solve these Related Problems:
- Write a C++ program that computes the sum of two integers and, if they are equal, returns three times the sum; otherwise, return their normal sum.
- Write a C++ program to add two numbers and output triple the sum only if both numbers are identical, using a ternary operator for selection.
- Write a C++ program that reads two integers, checks for equality, and then either prints the sum or triple the sum based on the result.
- Write a C++ program that computes the sum of two input values, and if they match, multiplies the sum by three before printing the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Basic Algorithm Exercises Home
Next: Write a C++ program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
What is the difficulty level of this exercise?