C++ Exercises: Compute the sum of values in a given array of integers except the number 17
Sum Array Elements Excluding 17
Write a C++ program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integers.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that calculates the sum of values in the array excluding the number 17
static int test(int nums[], int arr_length)
{
int sum = 0; // Initializing variable to store the sum
// Loop through the array to calculate the sum of values excluding 17
for (int i = 0; i < arr_length; i++)
{
if (nums[i] != 17) // Checking if the current number is not equal to 17
sum += nums[i]; // Adding the number to the sum if it's not 17
}
return sum; // Returning the sum of values excluding 17
}
int main()
{
int nums1[] = { 1, 5, 7, 9, 10, 17 }; // Initializing array nums1 with various integers including 17
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Displaying a message about the sum of values in the array excluding the number 17
cout << "Sum of values in the array of integers except the number 17:" << endl;
// Calling test function with nums1 and displaying the sum of values excluding 17
cout << test(nums1, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Sum of values in the array of integers except the number 17: 32
Visual Presentation:
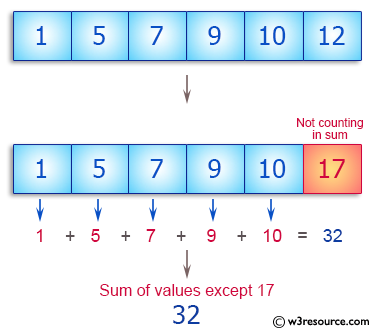
Flowchart:
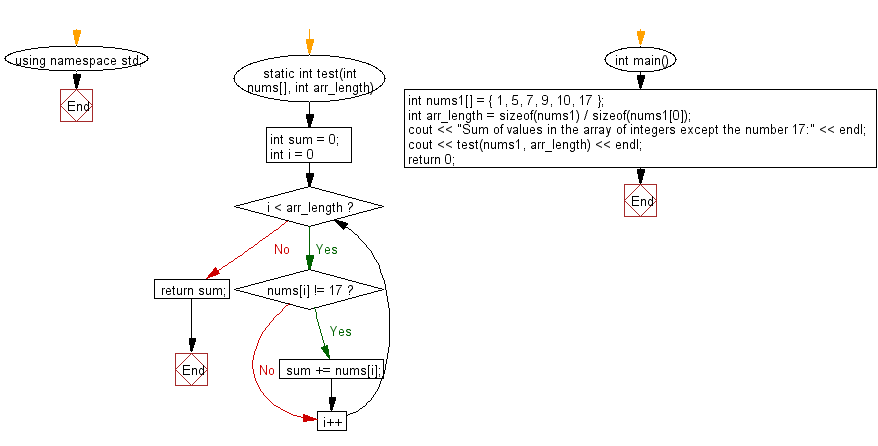
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of an array of integers while skipping any occurrence of the number 17.
- Write a C++ program that reads an array and sums all elements except those equal to 17, then outputs the total.
- Write a C++ program to calculate the aggregate of numbers in an array, ignoring the number 17, and print the result.
- Write a C++ program that processes an array and returns the sum of its elements, omitting any value that equals 17.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the difference between the largest and smallest values in a given array of integers and length one or more.
Next: Write a C++ program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?