C++ Exercises: Concatenate three copies of each string in a given list of strings
C++ Basic Algorithm: Exercise-127 with Solution
Write a C++ program to create a list from a given list of strings where each element is replaced by 3 copies of the string concatenated together.
Test Data:
Sample Input:
{ "1", "2", "3" , "4" }
Expected Output :
111 222 333 444
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard C++ libraries
#include <list> // Include the list container
using namespace std; // Using standard namespace
// Function to create a new list where each element is replaced by three copies of the string concatenated together
list<string> test(list<string> nums) {
list<string> new_list; // Declare a new list to store modified elements
list<string>::iterator it; // Declare an iterator for traversing the input list 'nums'
// Iterate through 'nums' list and concatenate three copies of each element together
for (it = nums.begin(); it != nums.end(); ++it) {
new_list.push_back(*it + *it + *it); // Concatenate three copies of the string
}
return new_list; // Return the new list with modified elements
}
// Function to display the elements of a list
display_list(list<string> g) {
list<string>::iterator it; // Declare an iterator for traversing the input list 'g'
// Iterate through 'g' list and display each element
for (it = g.begin(); it != g.end(); ++it) {
cout << *it << ' '; // Print each element followed by a space
}
cout << '\n'; // Move to a new line after displaying all elements
}
int main() {
list<string> text = { "1", "2", "3", "4", "5", "6" }; // Initialize a list 'text' with strings
cout << "Original list of elements:\n";
display_list(text); // Display the original list 'text'
list<string> result_list; // Declare a list to store the result of test() function
result_list = test(text); // Call the test() function to replace elements with three copies of the string
cout << "\nNew list from the said list where each element is replaced\nby 3 copies of the string concatenated together:\n";
display_list(result_list); // Display the new list with modified elements
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
Original list of elements: 1 2 3 4 5 6 New list from the said list where each element is replaced by 3 copies of the string concatenated together: 111 222 333 444 555 666
Flowchart:
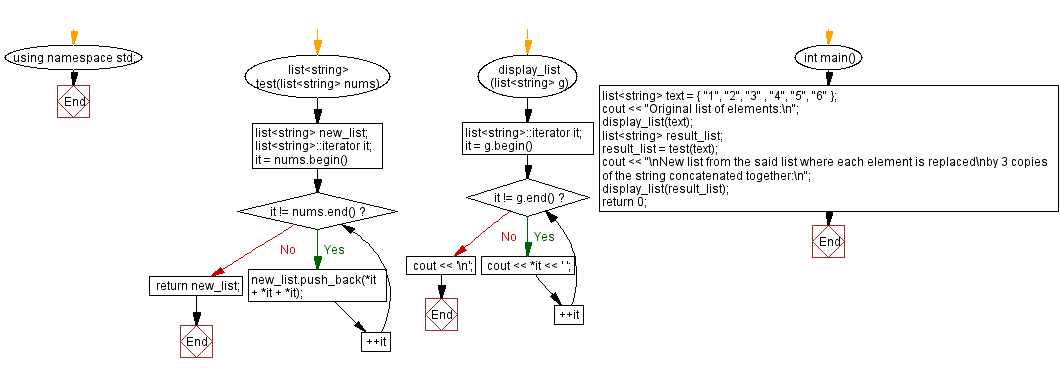
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: From a list of strings, create a new list with "$" at the beginning and end.
Next: Create a list of integers, add each value to 3 and multiply the result by 4.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics