C++ Exercises: Create a new string which is n copies of a given string
n Copies of String
Write a C++ program to create the string which is n (non-negative integer) copies of a given string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to concatenate a given string 's' 'n' times
string test(string s, int n)
{
string result = ""; // Initialize an empty string to store the result
// Loop 'n' times to concatenate the string 's' to the 'result' string
for (int i = 0; i < n; i++)
{
result += s; // Concatenate the string 's' to 'result'
}
return result; // Return the final concatenated string 'result'
}
// Main function
int main()
{
// Output the result of the test function with different input strings and numbers
cout << test("JS", 2) << endl; // Output: "JSJS"
cout << test("JS", 3) << endl; // Output: "JSJSJS"
cout << test("JS", 1) << endl; // Output: "JS"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
JSJS JSJSJS JS
Visual Presentation:
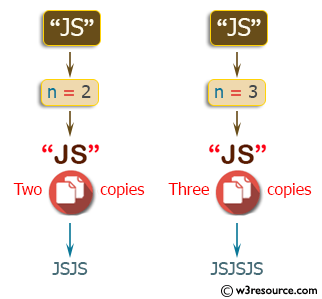
Flowchart:
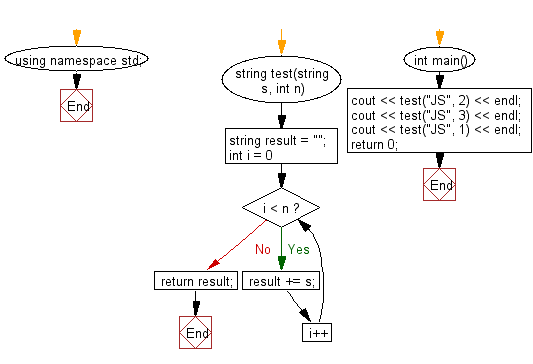
For more Practice: Solve these Related Problems:
- Write a C++ program to create a new string by concatenating n copies of an input string.
- Write a C++ program that reads a string and a non-negative integer n, then outputs the string repeated n times.
- Write a C++ program to generate a repeated string by looping n times and appending the original string during each iteration.
- Write a C++ program that takes a string and a repetition count and prints the concatenated result of the string repeated n times.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if two given non-negative integers have the same last digit.
Next: Write a C++ program to create a new string which is n (non-negative integer) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
What is the difficulty level of this exercise?