C++ Exercises: Create a new string from a given string where a specified character have been removed except starting and ending position of the given string
Remove Specified Character Except at Ends
Write a C++ program to create a new string from a given string where a specified character is removed except at the beginning and end.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to remove the second occurrence of a character from a string
string test(string str1, string c)
{
// Loop through the string starting from the second-to-last character
for (int i = str1.length() - 2; i > 0; i--)
{
// Check if the character at index 'i' in str1 is equal to the specified character 'c'
if (str1[i] == c[0])
{
// If found, remove the character at index 'i' from the string str1
str1 = str1.erase(i, 1);
}
}
return str1; // Return the modified string
}
int main()
{
// Test cases to remove the second occurrence of a specified character from a string
cout << test("xxHxix", "x") << endl;
cout << test("abxdddca", "a") << endl;
cout << test("xabjbhtrb", "b") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
xHix abxdddca xajhtrb
Visual Presentation:
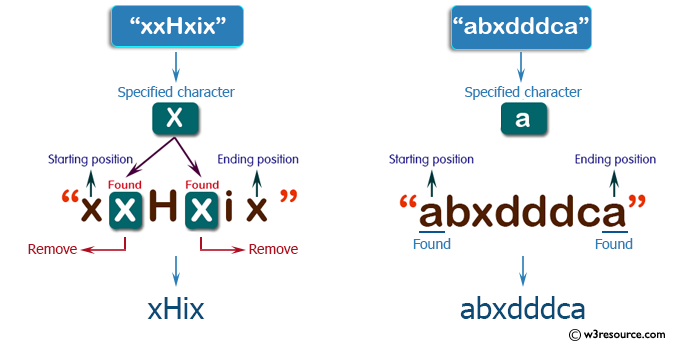
Flowchart:
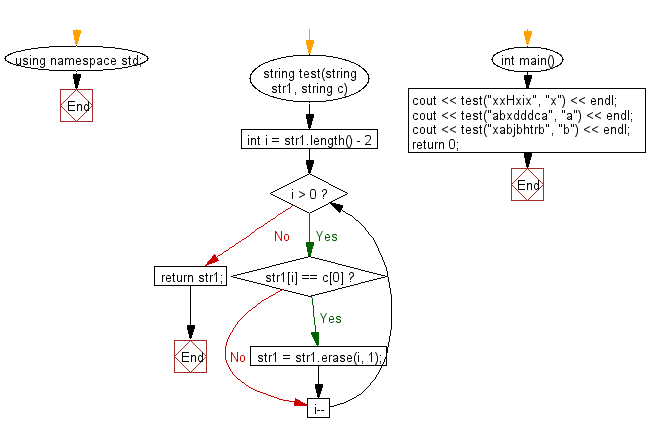
For more Practice: Solve these Related Problems:
- Write a C++ program to create a new string by removing all occurrences of a specified character except when it appears as the first or last character.
- Write a C++ program that reads a string and a character, then deletes that character from the string unless it is at either end.
- Write a C++ program to process a string and eliminate a given character from its middle portion, preserving the first and last characters.
- Write a C++ program that takes a string and a removal character, then outputs the string with that character removed from all positions except the boundaries.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array (length will be atleast 2) of integers and return true if there are two values 15, 15 next to each other.
Next: Write a C++ program to create a new string of the characters at indexes 0,1, 4,5, 8,9 ... from a given string.
What is the difficulty level of this exercise?