C++ Exercises: Check if three given numbers are in strict increasing order
Strict Increasing Order with Equality Option
Write a C++ program to check if three given numbers are in strict increasing order. For example, 4, 7, 15, or 45, 56, 67, but not 4 ,5, 8 or 6, 6, 8. However, if a fourth parameter is true, equality is allowed, such as 6, 6, 8 or 7, 7, 7.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes three integers (x, y, z) and a boolean flag as parameters
bool test(int x, int y, int z, bool flag)
{
// Returns based on the value of the flag:
// If flag is true, checks if x is less than or equal to y and y is less than or equal to z
// If flag is false, checks if x is less than y and y is less than z
return flag ? x <= y && y <= z : x < y && y < z;
}
int main()
{
// Testing the 'test' function with different sets of numbers and flags
cout << test(1, 2, 3, false) << endl; // Output: 1 (True, as 1 < 2 < 3)
cout << test(1, 2, 3, true) << endl; // Output: 1 (True, as 1 <= 2 <= 3)
cout << test(10, 2, 30, false) << endl; // Output: 0 (False, as 10 is not less than 2)
cout << test(10, 10, 30, true) << endl; // Output: 1 (True, as 10 <= 10 <= 30)
return 0;
}
Sample Output:
1 1 0 1
Visual Presentation:
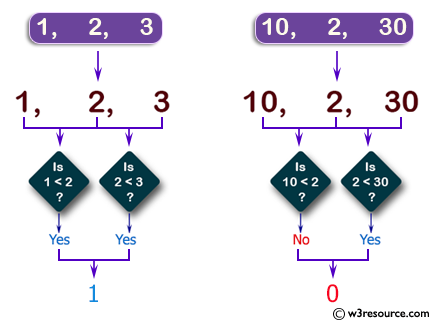
Flowchart:
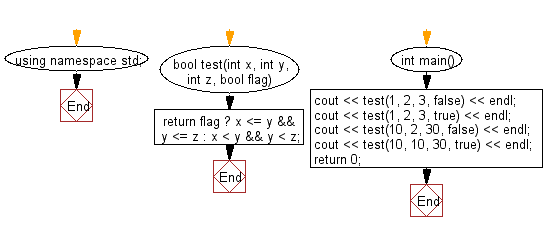
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if three integers are in strictly increasing order; if a fourth boolean parameter is true, allow equality between numbers.
- Write a C++ program that reads three numbers and an equality flag, then checks for ascending order, permitting equal values when allowed.
- Write a C++ program to compare three integers and return true for strict order, or allow duplicates if a condition parameter is set to true.
- Write a C++ program that tests if three input numbers are in increasing order, with an option to treat equal numbers as valid when a flag is provided.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if y is greater than x, and z is greater than y from three given integers x,y,z.
Next: Write a C++ program to check if two or more non-negative given integers have the same rightmost digit.
What is the difficulty level of this exercise?