C++ Exercises: Compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x
C++ Basic Algorithm: Exercise-51 with Solution
Sum with Same Number of Digits or Return x
Write a C++ program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x, return x without y.
Sample Solution:
C++ Code :
#include <iostream>
#include <string> // Included library for string handling
using namespace std;
// Function 'test' takes two integers (x, y) as parameters
int test(int x, int y)
{
// Converts the sum of x and y to a string and checks if its length
// is greater than the length of x converted to a string
// Returns x if the length of x is smaller than the length of x + y,
// otherwise returns the sum of x and y
return to_string(x + y).length() > to_string(x).length() ? x : x + y;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(4, 5) << endl;
cout << test(7, 4) << endl;
cout << test(10, 10) << endl;
return 0;
}
Sample Output:
9 7 20
Visual Presentation:
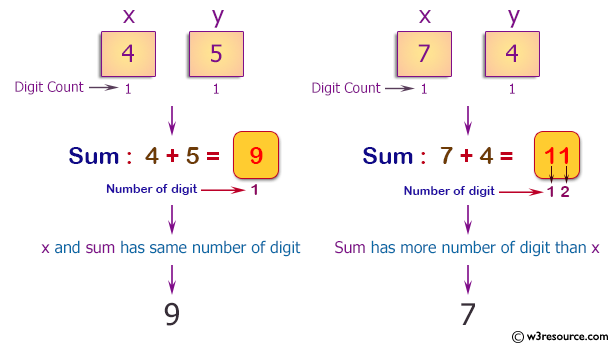
Flowchart:
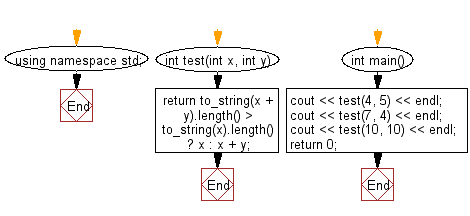
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33.
Next: Write a C++ program to compute the sum of three given integers. If the two values are same return the third value.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-51.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics