C++ Exercises: Compute the sum of the three given integers
C++ Basic Algorithm: Exercise-54 with Solution
Sum with 10–20 Treated as Zero Except 13/17
Write a C++ program to compute the sum of the three given integers. Except for 13 and 17, any value in the range 10..20 inclusive counts as 0.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Class Solution contains a function to manipulate numbers
class Solution
{
public:
// Function 'test' takes three integers (x, y, z) as parameters
int test(int x, int y, int z)
{
return fix_num(x) + fix_num(y) + fix_num(z); // Returns the sum after applying fix_num to each number
}
// Function 'fix_num' takes an integer 'n' as a parameter and processes it
int fix_num(int n)
{
// Checks if 'n' falls within certain ranges and replaces it with 0 if it does
return (n < 13 && n > 9) || (n > 17 && n < 21) ? 0 : n;
}
};
int main()
{
// Creating an instance of the Solution class
Solution *solution = new Solution();
// Testing the 'test' function with different sets of numbers
cout << solution->test(4, 5, 7) << endl;
cout << solution->test(7, 4, 12) << endl;
cout << solution->test(10, 13, 12) << endl;
cout << solution->test(17, 12, 18) << endl;
return 0;
}
Sample Output:
16 11 13 17
Visual Presentation:
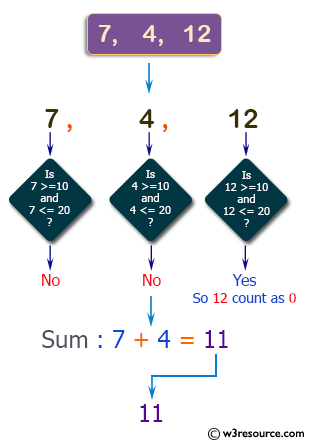
Flowchart:
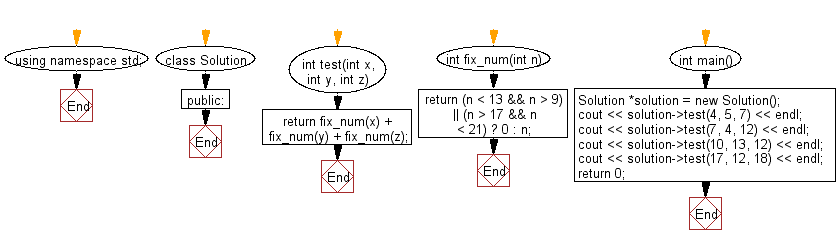
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Next: Write a C++ program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics