C++ Exercises: Check three given integers and return true if the difference between small and medium and the difference between medium and large is same
Equal Differences for Three Integers
Write a C++ program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' checks if the differences between the numbers are equal
bool test(int x, int y, int z)
{
// Checks for the condition when x is the largest number
if (x > y && x > z && y > z)
return x - y == y - z; // Returns true if differences are equal
// Checks for the condition when x is the largest number, but z is the smallest
if (x > y && x > z && z > y)
return x - z == z - y; // Returns true if differences are equal
// Checks for the condition when y is the largest number
if (y > x && y > z && x > z)
return y - x == x - z; // Returns true if differences are equal
// Checks for the condition when y is the largest number, but z is the smallest
if (y > x && y > z && z > x)
return y - z == z - x; // Returns true if differences are equal
// Checks for the condition when z is the largest number
if (z > x && z > y && x > y)
return z - x == x - y; // Returns true if differences are equal
// Returns true if differences are equal (when z is the largest number and y is the smallest)
return z - y == y - x;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(4, 5, 6) << endl;
cout << test(7, 12, 13) << endl;
cout << test(-1, 0, 1) << endl;
return 0;
}
Sample Output:
1 0 1
Visual Presentation:
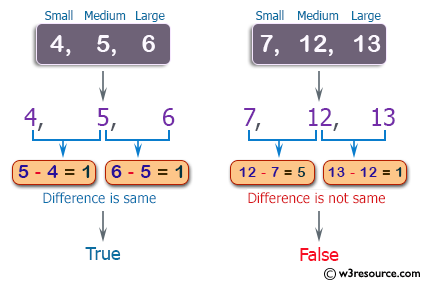
Flowchart:
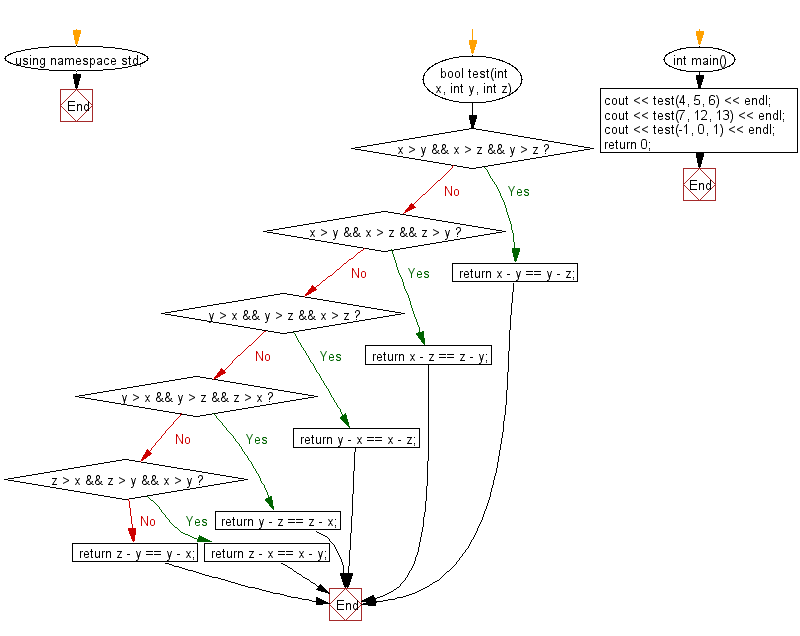
For more Practice: Solve these Related Problems:
- Write a C++ program to check if three integers are equally spaced, meaning the difference between the first and second equals the difference between the second and third.
- Write a C++ program that reads three numbers and returns true if the difference between the smallest and middle equals the difference between the middle and largest.
- Write a C++ program to verify whether three given integers have uniform differences between consecutive values, using subtraction operations.
- Write a C++ program that accepts three integer inputs and checks if they form an arithmetic progression by comparing consecutive differences.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Next: Write a C++ program to create a new string using two given strings s1, s2, the format of the new string will be s1s2s2s1.
What is the difficulty level of this exercise?