C++ Exercises: Insert a given string into middle of the another given string of length 4
Insert String in Middle of Another
Write a C++ program to insert a given string into middle of the another given string of length 4.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' modifies a string by inserting 'word' into 's1' at index 2
string test(string s1, string word)
{
// Concatenates the substring of 's1' from index 0 to 1, 'word', and the substring of 's1' from index 2 to the end
return s1.substr(0, 2) + word + s1.substr(2);
}
// Main function to test the 'test' function
int main()
{
// Displays the output of the 'test' function for different string inputs
cout << test("[[]]", "Hello") << endl; // Output: "[[Hello]]" (inserts "Hello" at index 2 of "[[]]")
cout << test("(())", "Hi") << endl; // Output: "((Hi))" (inserts "Hi" at index 2 of "(())")
return 0;
}
Sample Output:
[[Hello]] ((Hi))
Visual Presentation:
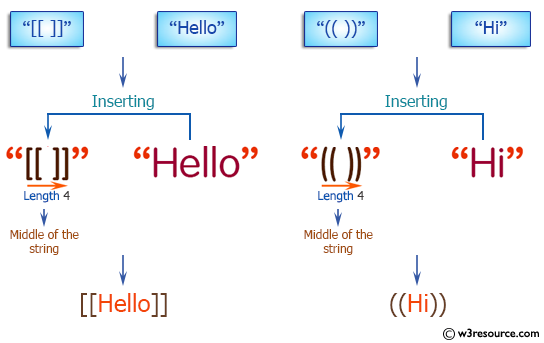
Flowchart:
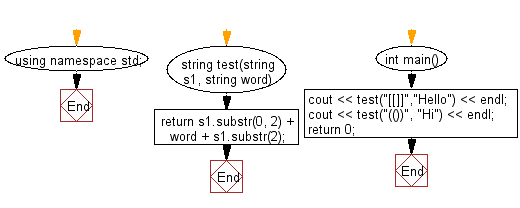
For more Practice: Solve these Related Problems:
- Write a C++ program to insert one string into the middle of another string of length 4 and output the new string.
- Write a C++ program that reads two strings and inserts the second string into the center of the first, assuming the first has a length of 4.
- Write a C++ program to take a four-character string and a second string, then output the result of inserting the second string exactly in the middle.
- Write a C++ program that accepts two strings and creates a new string by splitting the first in half and inserting the second between its halves.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string using two given strings s1, s2, the format of the new string will be s1s2s2s1.
Next: Write a C++ program to create a new string using three copies of the last two character of a given string of length atleast two.
What is the difficulty level of this exercise?