C++ Exercises: Check a given array of integers, length 3 and create a new array
Replace '7' with '1' if Preceded by '5'
Write a C++ program to check a given array of integers, length 3 and create an array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to modify the array nums by replacing '7' with '1' if preceded by '5'
int *test(int nums[], int arr_length) {
for (int i = 0; i < arr_length - 1; i++) {
if (nums[i] == 5 && nums[i + 1] == 7) // If the current element is '5' and the next element is '7'
nums[i + 1] = 1; // Replace '7' with '1'
}
return nums; // Return the modified array
}
// Main function
int main() {
int *p; // Declare a pointer to an integer
int nums1[] = {1, 5, 7}; // Define an array nums1
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculate the length of nums1
p = test(nums1, arr_length); // Call the test function with nums1
cout << "\nNew array: " << endl; // Output message for the new array
for (int i = 0; i < arr_length; i++) {
cout << *(p + i) << " "; // Output the elements of the modified array nums1
}
int nums2[] = {1, 5, 3, 7}; // Define another array nums2
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculate the length of nums2
p = test(nums2, arr_length); // Call the test function with nums2
cout << "\nNew array: " << endl; // Output message for the new array
for (int i = 0; i < arr_length; i++) {
cout << *(p + i) << " "; // Output the elements of the modified array nums2
}
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
New array: 1 5 1 New array: 1 5 3 7
Visual Presentation:
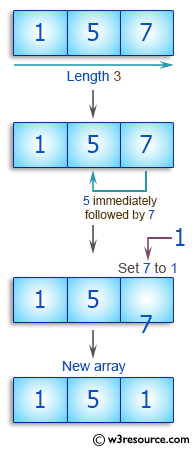
Flowchart:
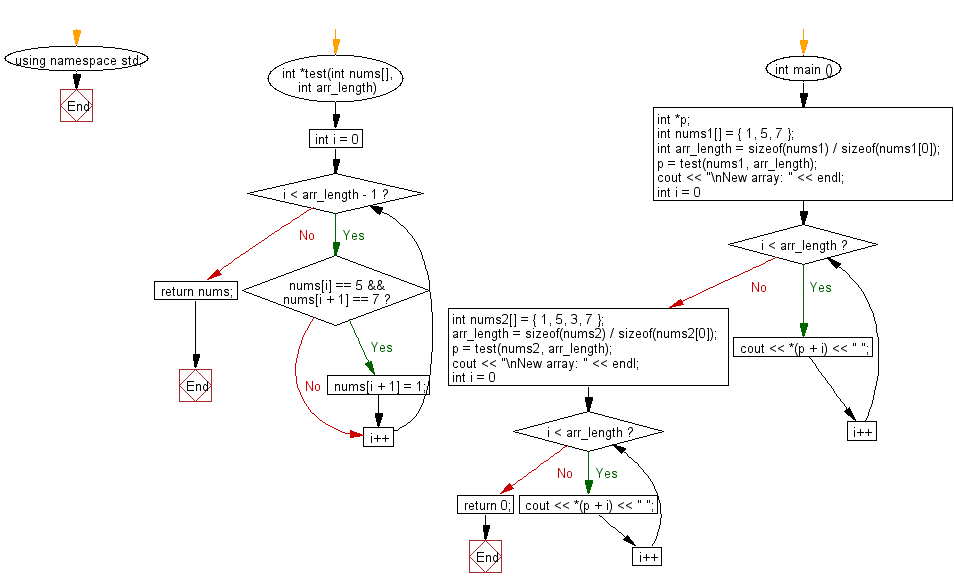
For more Practice: Solve these Related Problems:
- Write a C++ program to examine an array of integers and, if a 7 immediately follows a 5, replace the 7 with a 1.
- Write a C++ program that reads an integer array and modifies it so that any 7 following a 5 is substituted by 1, while leaving other elements unchanged.
- Write a C++ program to process an array and check for the pattern 5,7; if found, change the 7 to 1 and output the new array.
- Write a C++ program that iterates through an array and replaces any occurrence of 7 that is immediately after a 5 with the number 1.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.
Next: Write a C++ program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
What is the difficulty level of this exercise?