C++ Exercises: Print the result of the specified operations
Results of Specific Operations
Write a C++ program to print the results of the specified operations.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
cout << "\n\n Print the result of some specific operation :\n"; // Outputting a message indicating specific operations
cout << "--------------------------------------------------\n"; // Outputting a separator line
// Calculating and displaying results of specific mathematical expressions
cout << " Result of 1st expression is : "<< (-1+4*6) <<"\n" ; // Calculation: -1 + (4*6) = -1 + 24 = 23
cout << " Result of 2nd expression is : "<< ((35+5)%7) <<"\n" ; // Calculation: (35+5) % 7 = 40 % 7 = 5 (remainder of 40/7)
cout << " Result of 3rd expression is : "<< (14+-4*6/11) <<"\n" ; // Calculation: 14 - (4*6)/11 = 14 - 24/11 = 14 - 2 = 12
cout << " Result of 4th expression is : "<< (2+15/6*1-7%2) <<"\n\n" ; // Calculation: 2 + (15/6)*1 - (7%2) = 2 + 2 - 1 = 4 - 1 = 3
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Print the result of some specific operation : -------------------------------------------------- Result of 1st expression is : 23 Result of 2nd expression is : 5 Result of 3rd expression is : 12 Result of 4th expression is : 3
Flowchart:
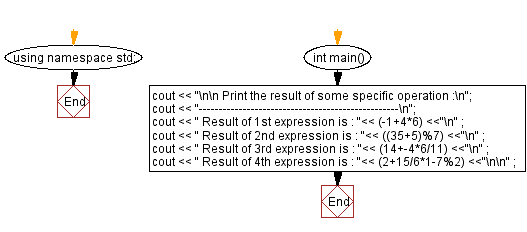
For more Practice: Solve these Related Problems:
- Write a C++ program to evaluate multiple arithmetic expressions and print each result with a label.
- Write a C++ program that computes a series of expressions and formats the results in a clear, columnar layout.
- Write a C++ program to compute a complex expression step-by-step and display the intermediate and final results.
- Write a C++ program that allows the user to select an expression from a list and then computes and displays its result.
C++ Code Editor:
Previous: Write a program in C++ to formatting the output.
Next: Write a program in C++ to add two numbers accept through keyboard.
What is the difficulty level of this exercise?