C++ Exercises: Convert temperature in Celsius to Fahrenheit
Celsius to Fahrenheit Conversion
Write a C++ program to convert temperature in Celsius to Fahrenheit.
Visual Presentation:
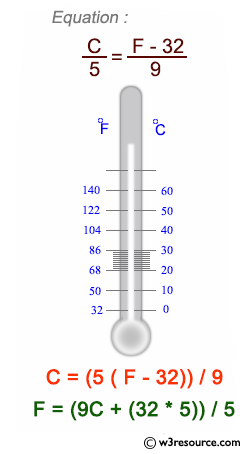
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream header file
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
float frh, cel; // Declaring floating-point variables for Fahrenheit and Celsius temperatures
cout << "\n\n Convert temperature in Celsius to Fahrenheit :\n"; // Outputting a message indicating temperature conversion
cout << "---------------------------------------------------\n"; // Outputting a separator line
cout << " Input the temperature in Celsius : "; // Prompting the user to input the temperature in Celsius
cin >> cel; // Taking input for the Celsius temperature from the user
frh = (cel * 9.0) / 5.0 + 32; // Converting Celsius to Fahrenheit using the formula: (Celsius * 9/5) + 32
cout << " The temperature in Celsius : " << cel << endl; // Displaying the input temperature in Celsius
cout << " The temperature in Fahrenheit : " << frh << endl; // Displaying the converted temperature in Fahrenheit
cout << endl; // Outputting a blank line for better readability
return 0; // Returning 0 to indicate successful program execution
} // End of the main function
Sample Output:
Convert temperature in Celsius to Fahrenheit : --------------------------------------------------- Input the temperature in Celsius : 35 The temperature in Celsius : 35 The temperature in Fahrenheit : 95
Flowchart:
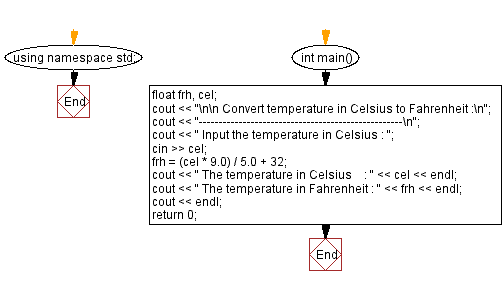
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a temperature from Celsius to Fahrenheit and then back to Celsius to check for consistency.
- Write a C++ program that accepts a temperature in Celsius, converts it to Fahrenheit, and prints both values with appropriate labels.
- Write a C++ program to convert Celsius to Fahrenheit using a function and then display the result in a formatted table.
- Write a C++ program that converts a list of Celsius temperatures to Fahrenheit and prints the conversion in a loop.
C++ Code Editor:
Previous: Write a program in C++ to find the area and circumference of a circle.
Next: Write a program in C++ to convert temperature in Fahrenheit to Celsius.
What is the difficulty level of this exercise?