C++ Exercises: Swap first and last digits of any number
Swap First and Last Digit
Write a C++ program to swap the first and last digits of any number.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <math.h> // Including math functions header file
using namespace std; // Using the standard namespace
int main() { // Start of the main function
int n, first, last, sum, digits, nn, a, b; // Declaration of integer variables
cout << "\n\n Find the number after swapping the first and last digits:\n"; // Displaying program's purpose
cout << "-------------------------------------------------------------\n";
cout << " Input any number: ";
cin >> n; // Taking input from the user
digits = (int)log10(n); // Calculating the number of digits in the number
// Extracting the first and last digits of the number
first = n / pow(10, digits); // Getting the first digit
last = n % 10; // Getting the last digit
// Swapping the first and last digits
a = first * (pow(10, digits)); // Multiplying the first digit by a power of 10
b = n % a; // Getting the remaining digits after removing the first digit
n = b / 10; // Removing the last digit
// Constructing the new number after swapping
nn = last * (pow(10, digits)) + (n * 10 + first); // Constructing the new number
cout << " The number after swapping the first and last digits is: " << nn << endl; // Displaying the result
}
Sample Output:
Find the number after swapping the first and last digits: ------------------------------------------------------------- Input any number: 12345 The number after swaping the first and last digits are: 52341
Flowchart:
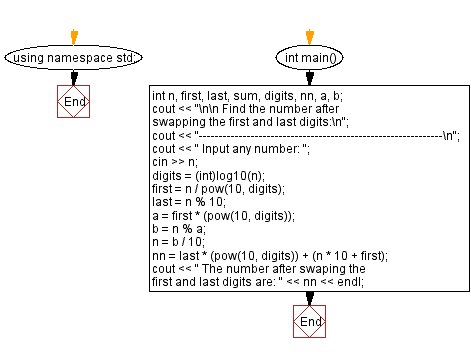
For more Practice: Solve these Related Problems:
- Write a C++ program that swaps the first and last digits of a number without converting it to a string.
- Write a C++ program to swap the first and last digits of an integer using arithmetic operations and modulus.
- Write a C++ program that reads a number and swaps its first and last digits, ensuring correct behavior for single-digit numbers.
- Write a C++ program to swap the first and last digits of a number and then check if the new number is a palindrome.
C++ Code Editor:
Previous: Write a program in C++ to add two binary numbers.
Next: Write a C++ program to which reads an given integer n and prints a twin prime which has the maximum size among twin primes less than or equals to n.
What is the difficulty level of this exercise?