C++ Exercises: Add all the numbers from 1 to a given number
Sum from 1 to n
Pictorial Presentation:
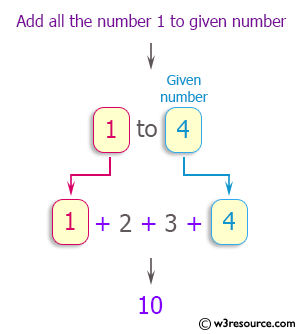
Write a C++ program to add all the numbers from 1 to a given number.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using the standard namespace
// Function to calculate the sum of numbers from 1 to n
int Add_1_to_given_number(int n) {
int total = 0; // Variable to store the total sum
// Loop to add numbers from 1 to n
for (int x = 1; x <= n; x++) {
total += x; // Adding each number to the total sum
}
return total; // Returning the final total sum
}
int main() {
// Printing the sum of numbers from 1 to 4 by calling the Add_1_to_given_number function with argument 4
cout << "\nAdd 1 to 4: " << Add_1_to_given_number(4);
// Printing the sum of numbers from 1 to 100 by calling the Add_1_to_given_number function with argument 100
cout << "\nAdd 1 to 100: " << Add_1_to_given_number(100);
return 0; // Indicating successful completion of the program
}
Sample Output:
Add 1 to 4: 10 Add 1 to 100: 5050
Flowchart:
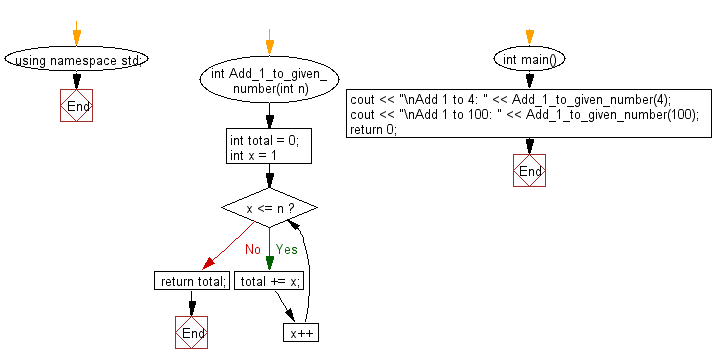
For more Practice: Solve these Related Problems:
- Write a C++ program that computes the sum of all numbers from 1 to n using a recursive function and compares it to the formula-based result.
- Write a C++ program to calculate the sum from 1 to n using a loop and then display the result in a formatted equation.
- Write a C++ program that computes the sum from 1 to n and then determines if the result is a prime number.
- Write a C++ program to calculate the sum of numbers from 1 to n, then compute the average of these numbers, and display both results.
C++ Code Editor:
Previous: Write a C++ program to check whether given length of three side form a right triangle.
Next: Write a C++ program to which prints the central coordinate and the radius of a circumscribed circle of a triangle which is created by three points on the plane surface.
What is the difficulty level of this exercise?