C++ Exercises: Compute the difference between the highest number and the lowest number
Difference Between Max and Min Numbers
Write a C++ program that accepts various numbers and computes the difference between the highest number and the lowest number. All input numbers should be real numbers between 0 and 1,000,000. The output (real numbers) may include an error of 0.01 or less.
Visual Presentation:
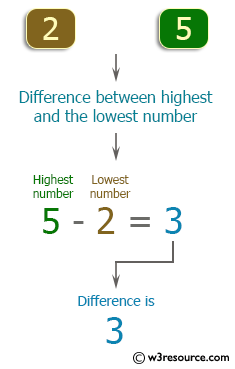
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream operations
using namespace std;
int main()
{
double num[52]; // Array to store up to 52 double numbers
int c = 0; // Counter variable initialized to 0
// Loop to read numbers from input and store them in the 'num' array
while (cin >> num[c++]); // Reads numbers until invalid input is encountered and increments the counter 'c'
sort(num, num + c - 1); // Sorting the array of numbers
// Displaying the difference between the highest and lowest numbers entered
cout << "Difference between the highest number and the lowest number: ";
cout << num[c - 2] - num[0] << endl; // Output the difference between the highest and lowest numbers
return 0; // Indicating successful completion of the program
}
Sample Output:
Sample Input: 2 5 Difference between the highest number and the lowest number: 3
Flowchart:
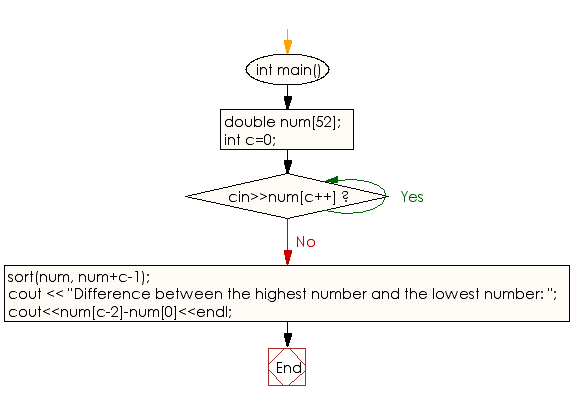
For more Practice: Solve these Related Problems:
- Write a C++ program to read a list of real numbers and then compute the difference between the highest and lowest values using loops.
- Write a C++ program that accepts floating-point numbers, determines the maximum and minimum, and then calculates their difference with error tolerance.
- Write a C++ program to compute the difference between the maximum and minimum values in an array without using built-in functions.
- Write a C++ program that finds the max and min numbers in a dynamically allocated array and then prints the difference with two decimal precision.
C++ Code Editor:
Previous: Write a C++ program that accepts sales unit price and sales quantity of various items and compute total sales amount and the average sales quantity. All input values must greater than or equal to 0 and less than or equal to 1,000, and the number of pairs of sales unit and sales quantity does not exceed 100. If a fraction occurs in the average of the sales quantity, round the first decimal place.
Next: Write a C++ program to compute the sum of the specified number of Prime numbers.
What is the difficulty level of this exercise?